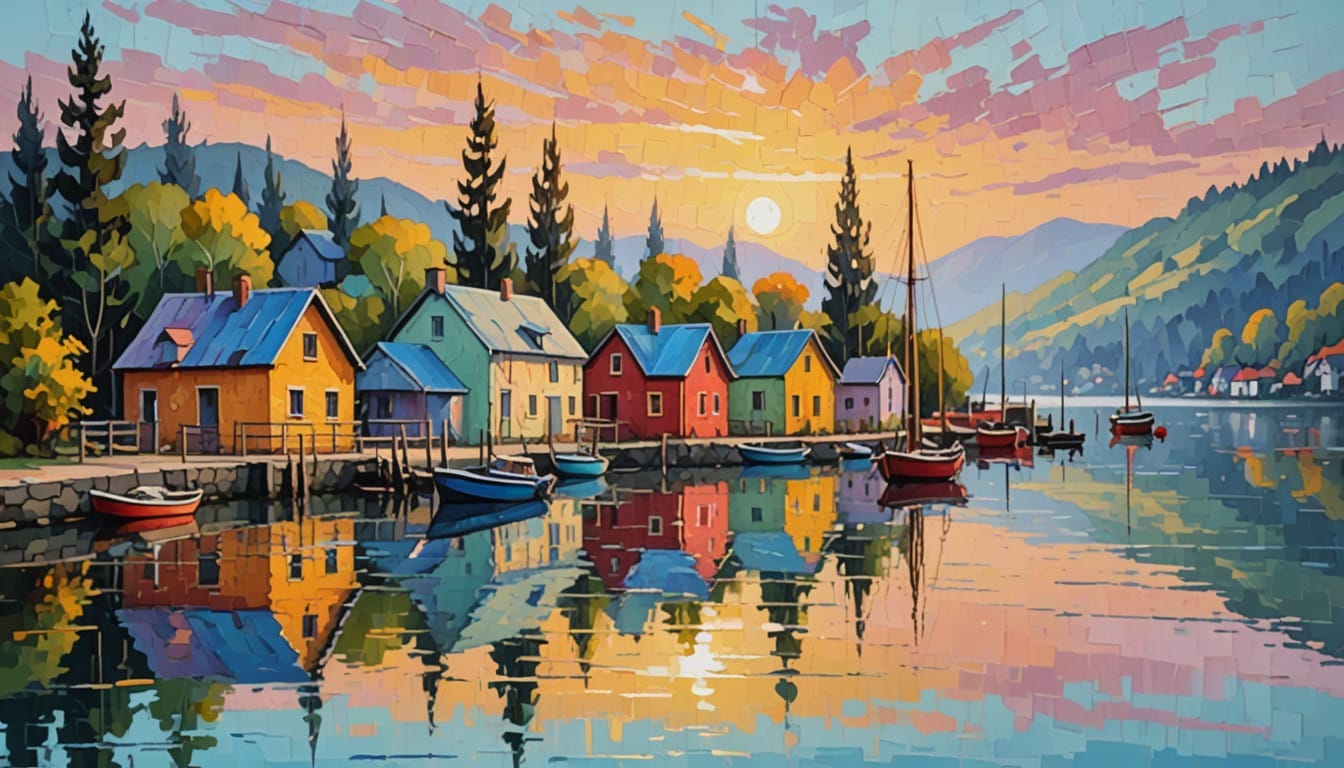
So, you want to build your first React app and deploy it on AWS? You’re in the right place. Let’s create a simple “Hello, World!” app using AWS Lambda, S3, and CloudFront, while keeping it all beginner-friendly (and mildly entertaining).
Step 1: Prerequisites (Don’t Skip This)
Before we dive in, here’s what you’ll need:
- An AWS account. If you don’t have one yet, head over to AWS and sign up.
- Node.js installed on your machine. Get it here.
- Basic knowledge of React and terminal commands (or a willingness to learn via trial and error).
- Coffee. Optional but highly recommended.
Step 2: Create a React App
Open your terminal and run the following command to create a new React app:
npm create vite@latest hello-world --template react-ts
This sets up a new React app in a folder called hello-world
. Navigate into that folder:
cd hello-world
Feel free to edit the src/App.tsx
file to replace the boilerplate code with a simple “Hello, World!”:
function App() {
return (
<div>
<h1>Hello, World!</h1>
</div>
);
}
export default App;
Run npm run dev
to preview your masterpiece in the browser. If it works, great—you’re halfway to fame.
Step 3: Prepare Your App for Production
Time to build your app so it’s production-ready:
npm run build
This creates a build
folder containing your app’s static files.
Step 4: Set Up an S3 Bucket
- Log in to your AWS Management Console and go to S3.
- Create a bucket. Give it a unique name, like
my-react-app-bucket
. (Pro tip: Avoid spaces in bucket names unless you like debugging.) - Enable public access for the bucket via the Permissions tab. Check the ‘Block Public Access’ settings and disable ‘Block all public access’. Then, configure your bucket policy properly. Go to your S3 bucket’s permissions tab, click on ‘Bucket policy,’ and paste a JSON policy like this:
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Principal": "*",
"Action": "s3:GetObject",
"Resource": "arn:aws:s3:::your-bucket-name/*"
}
]
}
Replace your-bucket-name
with the actual name of your bucket. This enables public read access to the objects inside while keeping the bucket itself secure.
4. Upload the contents of your dist
folder to the bucket.
Step 5: Configure S3 for Static Website Hosting
- In your bucket settings, go to the Properties tab.
- Enable “Static website hosting.”
- Set the index document to
index.html
.
Your app is now technically live! You can grab the bucket’s website endpoint and view your app.
Step 6: Add CloudFront for Distribution
To make your app blazing fast and secure:
- Go to the CloudFront service in the AWS console.
- Create a new distribution and set your S3 bucket as the origin.
- Once deployed, CloudFront will give you a URL to access your app.
Voilà! Your app is now running on AWS — and looking good while doing it.
Step 7: Bonus Points with Lambda (Optional)
Want to add some backend magic? AWS Lambda is your friend:
- Go to the Lambda service in AWS.
- Create a function (Node.js runtime).
- Write a simple handler that says hello:
exports.handler = async (event) => {
return {
statusCode: 200,
body: "Hello from Lambda!",
};
};
4. Deploy your function and connect it to an API Gateway endpoint. To do this, go to the API Gateway service in the AWS Management Console, create a new HTTP API, and link it to your Lambda function. To do this, navigate to the API Gateway service in the AWS Console, select ‘Create API,’ and choose an HTTP API. During the creation process, select the ‘Integrations’ section, add a Lambda function integration, and choose your deployed function. Next, under ‘Routes,’ add a route (e.g., /hello
) and connect it to the Lambda integration. Deploy the API to a stage (e.g., ‘default’) to get a public URL for testing.. Under ‘Routes,’ define a route for your endpoint (e.g., /hello
) and map it to the Lambda integration. Deploy your API and note the endpoint URL provided. Test the URL in your browser or a tool like Postman to ensure your Lambda function responds as expected.
Now your React app can call the Lambda function via the API Gateway. Fancy!
Step 8: Bask in Your Glory
Congratulations! You’ve just deployed your first React app on AWS. Reward yourself with something indulgent, like a snack or a well-earned nap.
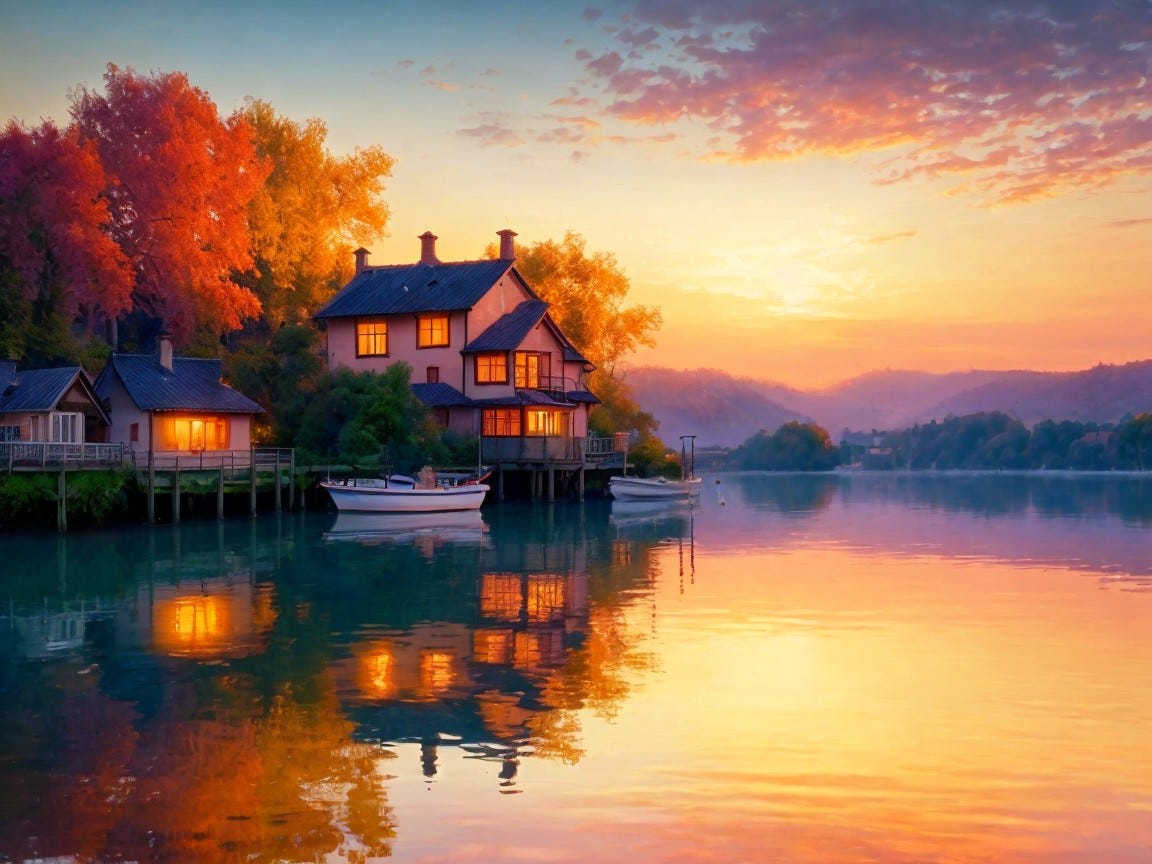
Art Prompt
Create an impressionist-style digital painting of a serene lakeside village at sunrise, with softly blurred edges, warm pastel tones, and reflections in the still water. Include small boats docked by wooden piers and subtle hints of morning activity, such as villagers walking along a cobblestone path.
Did you find this guide helpful? Confusing? Funny? Let me know in the comments! Don’t forget to follow me for more cloud chaos and coding tips.