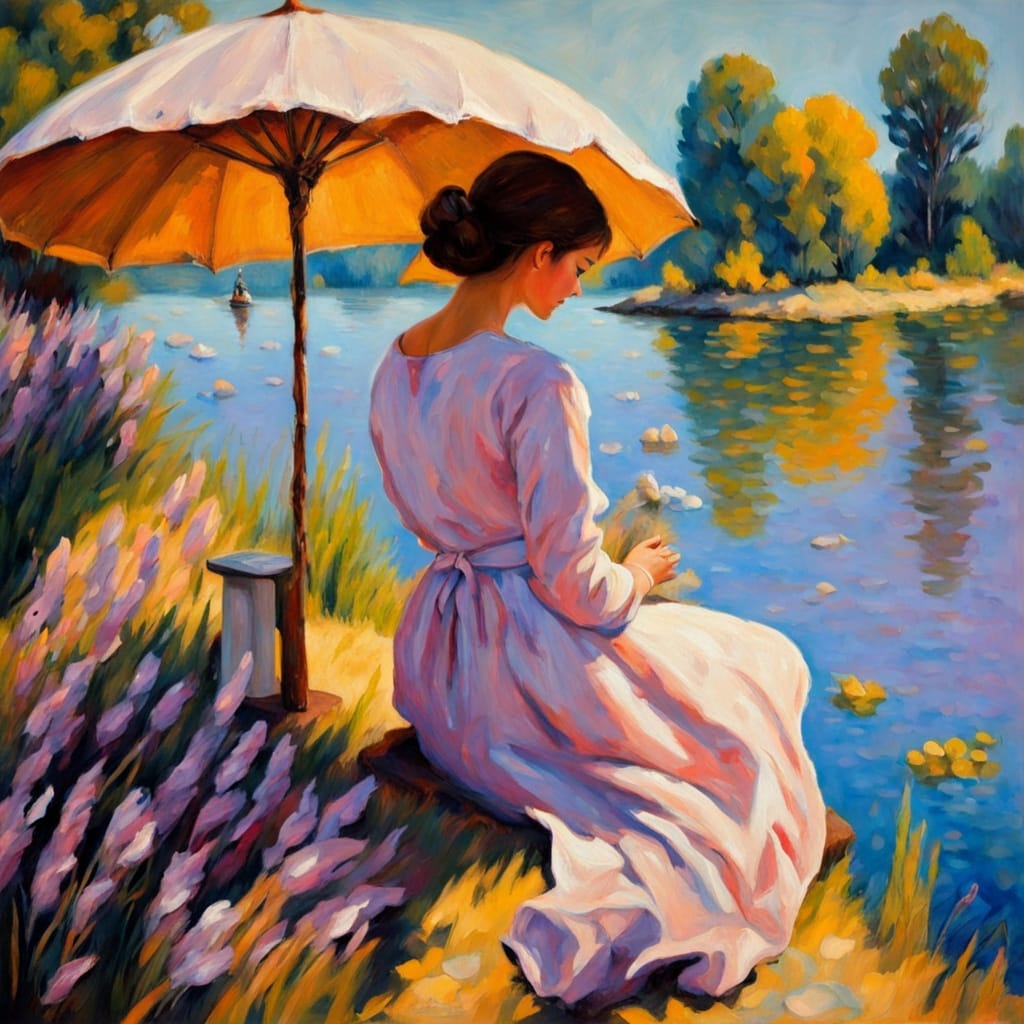
Let’s say you’re building an app. It’s going to be the next big thing. Bigger than TikTok but for spreadsheets. You need to store data, but raw SQL makes you feel like you’re defusing a bomb with chopsticks. Enter SQLAlchemy, the lovechild of Python and databases that says, “Hey, what if we made querying data not feel like medieval torture?”
So… What Is SQLAlchemy?
SQLAlchemy is Python’s most popular Object Relational Mapper (ORM). It lets you interact with databases like you’re talking to Python objects rather than manually crafting cryptic SQL queries like a wizard in an IT dungeon.
At its core, SQLAlchemy gives you:
- A full-blown ORM if you’re into that high-level abstraction life.
- A SQL Expression Language if you like to keep it raw (but not too raw).
- A way to flip the database table into Python objects and back, kind of like Pokémon evolutions but for data.
Homepage: https://www.sqlalchemy.org
Is It Still Relevant or Is This Just Another ’00s Relic?
SQLAlchemy isn’t just still relevant — it’s thriving. Like that one friend who still uses a flip phone and somehow makes it work. Major Python frameworks like Flask and FastAPI have it basically baked in. It’s in active development and gets love from everyone who’s ever screamed at a malformed SQL JOIN.
Pros, Cons, and Mild Existential Crises
Pros:
- Clean, elegant abstraction of databases.
- Optional deep dive into SQL if you’re feeling bold.
- Integrates with everything from Flask to FastAPI to Alembic for migrations.
- Massive community and robust documentation.
Cons:
- Steeper learning curve than other ORMs (looking at you, Django).
- Complexity increases fast if you go off the beaten path.
- Sometimes you will write more code than raw SQL for the same result. But hey, it’s readable!
Use Cases: AKA “When Should I Invite This Nerd to the Party?”
- Building a Python web app (Flask, FastAPI).
- Scripting batch data jobs.
- Querying, inserting, and manipulating data without managing SQL strings manually.
- Teaching someone how databases work without breaking their soul.
A Little Example to Make You Go “Ohhhhhh”
from sqlalchemy import Column, Integer, String, create_engine
from sqlalchemy.orm import declarative_base, sessionmaker
Base = declarative_base()
class Sandwich(Base):
__tablename__ = 'sandwiches'
id = Column(Integer, primary_key=True)
name = Column(String)
bread = Column(String)
engine = create_engine('sqlite:///lunch.db')
Base.metadata.create_all(engine)
Session = sessionmaker(bind=engine)
session = Session()
session.add(Sandwich(name="BLT", bread="Sourdough"))
session.commit()
Boom. That’s a sandwich. In a database. You’re a data chef now.
History and Fame: This Ain’t Its First Rodeo
SQLAlchemy was first released in 2006 by Mike Bayer, who probably deserves a Nobel Peace Prize for stopping countless SQL meltdowns. It’s matured over nearly two decades without turning into a bloated monster. It’s like the Linux of ORMs — reliable, geek-approved, and surprisingly sleek when styled right.
Alternatives (aka the Frenemies)
- Django ORM — Simpler, tightly integrated with Django, but less flexible.
- Peewee — Lightweight ORM for people who like minimalism and tiny furniture.
- Tortoise ORM — Async-focused, plays nice with FastAPI.
- Raw SQL — For when you’re feeling lucky, punk.
Popularity: Hot or Not?
SQLAlchemy is hot like a jalapeño in July. According to Stack Overflow’s Developer Survey, SQLAlchemy consistently ranks among the top Python libraries in backend web development. It’s a staple in the Python tech stack — not as flashy as TensorFlow, but 1000x more likely to keep your app from imploding.
It peaked in popularity around 2020 (blame pandemic hobby projects), but it remains very much on-trend.
Tech Stack Compatibility: Basically Everyone’s Friend
SQLAlchemy works with:
- PostgreSQL
- MySQL
- SQLite
- Oracle (if you’re into pain)
- MSSQL
And it plays nice with:
- Flask
- FastAPI
- Pandas (with
.to_sql
) - Alembic (for migrations)
- Jupyter notebooks
- AI workflows where persistence matters
So yes, it even gets along with AI. Store embeddings, logs, prompt history, or anything else your LLM dreams up. Just don’t try to store actual sentience in SQLite. It gets jealous.
Weird Tidbit: Is It the Subject of Famous Art?
Not yet. But imagine a classical oil painting of a Roman philosopher holding up an ERD diagram like it’s the Ten Commandments. SQLAlchemy would be that diagram.
Companies Using It (aka The Cool Kids Table)
- OpenAI
- Lyft
- Yelp
- NASA (yep)
- Any Python developer who’s said “I hate SQL, but not that much”
Final Thoughts
SQLAlchemy is that loyal, slightly nerdy friend who remembers your coffee order, helps you debug at 3AM, and doesn’t judge when your schema looks like spaghetti. It’s not always the easiest to learn, but once you do — it’s pure magic.
If you’ve used it and have thoughts (or strong sandwich opinions), drop a comment. And hey — hit that follow button if you want more spicy takes on Python tools, AI oddities, and the occasional programming therapy session.
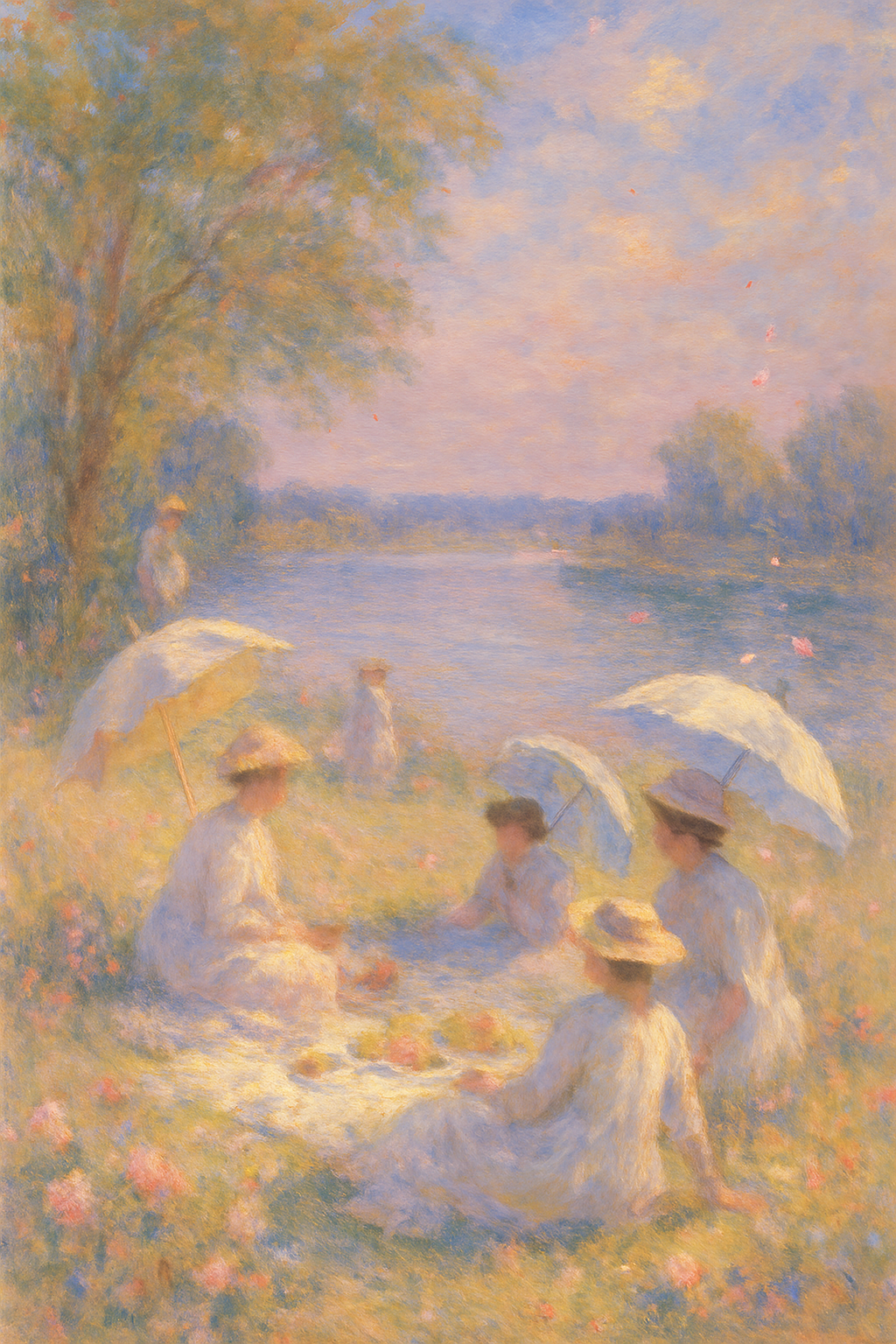
Art Prompt:
A sun-drenched picnic unfolds on the edge of a quiet lake, where soft, shimmering water reflects the blush of a lavender sky. Figures in flowing white garments lounge beneath parasols, their features blurred into serenity by gentle brush strokes reminiscent of Renoir. Loose petals drift on the breeze like whispered memories, while the landscape glows in pastels of peach, coral, and pale blue. The entire scene is a moment of dreamlike stillness, caught between laughter and silence.