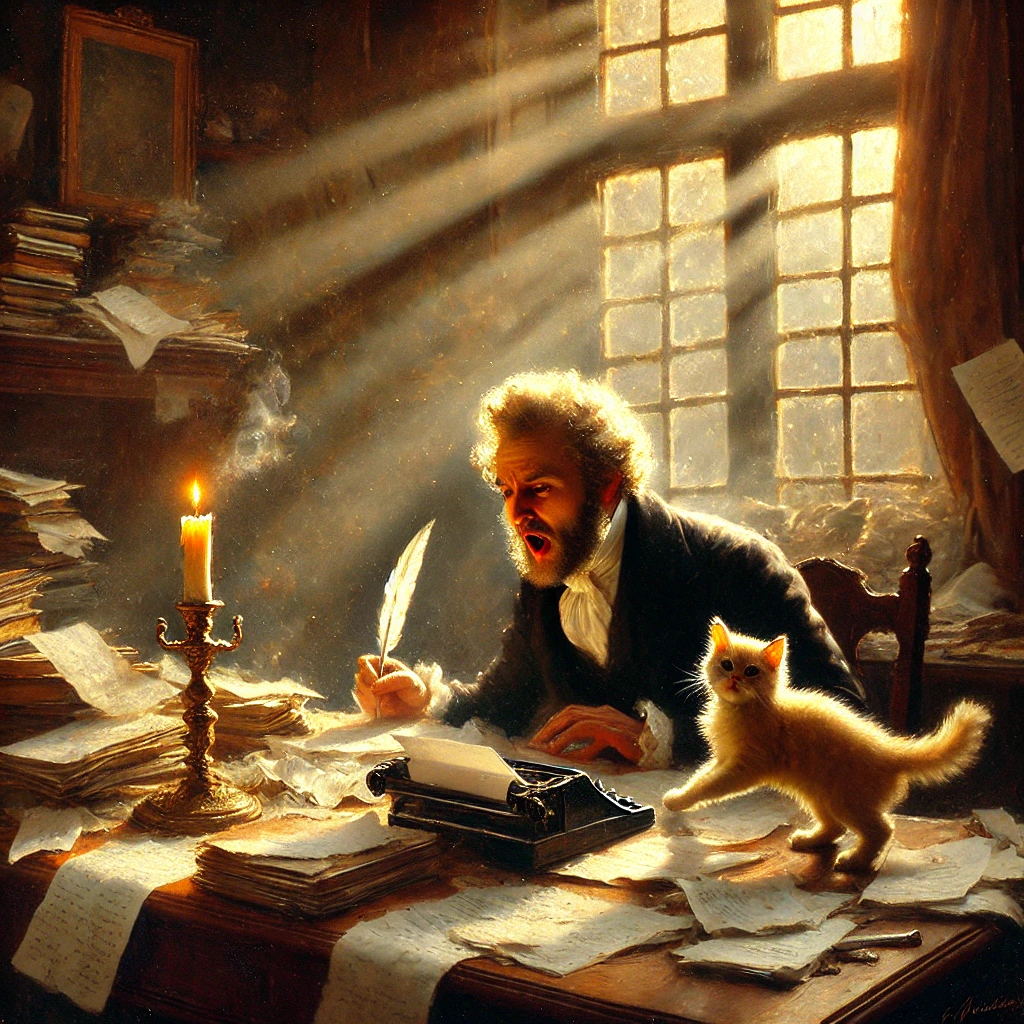
If you’ve ever written Python code that involves user input, APIs, or databases, you’ve probably spent way too much time validating data. You start out thinking, “Eh, it’s just a string, how bad could it be?” Then one fateful day, your function chokes on an unexpected None
, a rogue integer infiltrates your email field, and you spiral into an existential crisis.
Enter Pydantic, the unsung hero of data validation, ready to save your sanity one type-checked field at a time.
What Is Pydantic?
Pydantic is a Python library that makes data validation feel less like wrestling an angry octopus and more like sipping a well-organized cup of coffee. It’s a tool that lets you define data models with type hints and then automatically checks incoming data for compliance. If something is off, Pydantic will call it out like a strict but fair high school English teacher.
For example, let’s say you’re building an app where users input their name, age, and email:
from pydantic import BaseModel, EmailStr
class User(BaseModel):
name: str
age: int
email: EmailStr
user = User(name="Alice", age=25, email="alice@example.com") # Works fine
user = User(name="Bob", age="not-a-number", email="bob@example.com") # Throws an error
Boom. No need to manually write if isinstance(age, int)
nonsense. Pydantic takes care of it.
Is It Still Relevant?
Oh, absolutely. If anything, Pydantic has only grown in importance as Python applications become more complex. With FastAPI, a popular web framework that basically treats Pydantic as its BFF, data validation has become almost effortless. In an era where bad data can break your application faster than a toddler with an iPad, Pydantic is an essential tool in any Python developer’s belt.
Pros and Cons
✅ Pros:
- Automatic validation: No more
if
statements everywhere. - Great error messages: Tells you exactly what went wrong.
- Type hints make it readable: Your IDE will love you.
- Works out of the box with FastAPI.
- Supports JSON serialization and deserialization.
- It’s fast (Pydantic v2 is even faster thanks to Rust-powered internals).
❌ Cons:
- Can be overkill for tiny projects.
- Learning curve if you’re not used to type hints.
- If your data structures are overly complex, model nesting can get intense.
How Popular Is Pydantic?
It’s thriving. It has over 15K stars on GitHub and is used by companies like Microsoft, Uber, and Explosion AI (the creators of spaCy). Its popularity skyrocketed with FastAPI’s rise, since FastAPI leans heavily on Pydantic for request validation.
Who Invented It?
Pydantic was created by Samuel Colvin, a developer who clearly got tired of dealing with messy, unvalidated data and decided to do something about it. A true hero.
What Are the Alternatives?
If Pydantic isn’t quite your vibe, you might consider:
- Marshmallow: Older and more serialization-focused.
- Attrs: Focuses more on data classes than validation.
- Django Models: If you’re in Django-land, this might already be your thing.
But let’s be real — Pydantic is the best at what it does.
Does It Work Well With AI?
Actually, yes! If you’re working with AI models that output structured data (like OpenAI’s function calling), you can use Pydantic to validate that output before your app chokes on something unexpected.
class AIResponse(BaseModel):
intent: str
confidence: float
response = AIResponse(intent="greeting", confidence=0.98)
What Tech Stack Does It Work With?
Pydantic plays nice with:
- FastAPI (its #1 fan)
- SQLAlchemy (for ORM integration)
- Databases (when paired with async models)
- Machine Learning APIs (for response validation)
- Anything Pythonic that benefits from structured data
Final Thoughts
If you’re not using Pydantic yet, you’re making your life harder than it needs to be. Whether you’re building APIs, working with user inputs, or managing structured data, Pydantic is the sidekick you need. Try it out, and let me know in the comments how it saved you from a data disaster!
Follow me for more Python adventures, and drop a comment below — especially if Pydantic has ever saved your bacon.
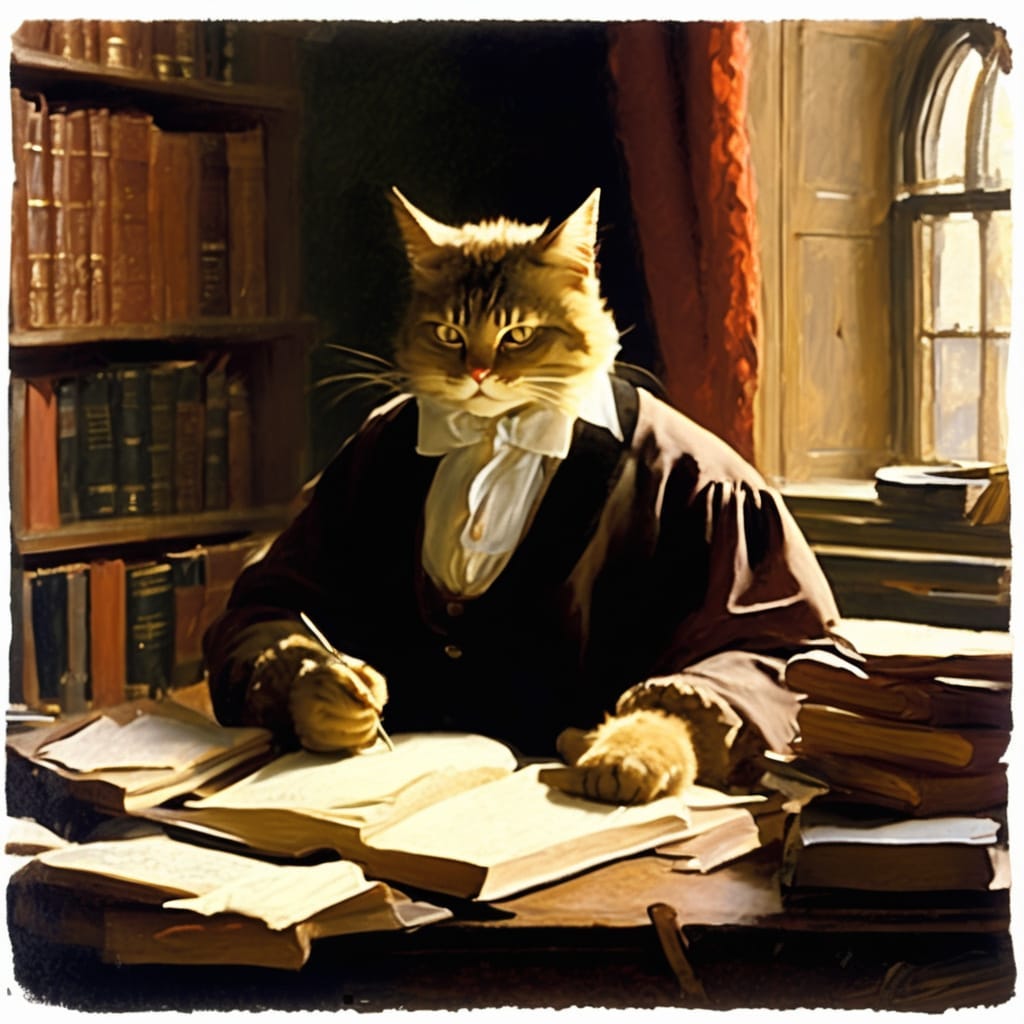
Art Prompt:
A 19th-century Impressionist painting of a scholar in a sunlit study, surrounded by scattered papers and open books, feverishly writing notes while a small, mischievous cat bats at the quill. The brushstrokes capture the flickering candlelight on the wooden desk and the hazy afternoon glow filtering through a grand window. The scholar’s expression is both exasperated and amused, as though wrestling with a particularly stubborn data validation error.