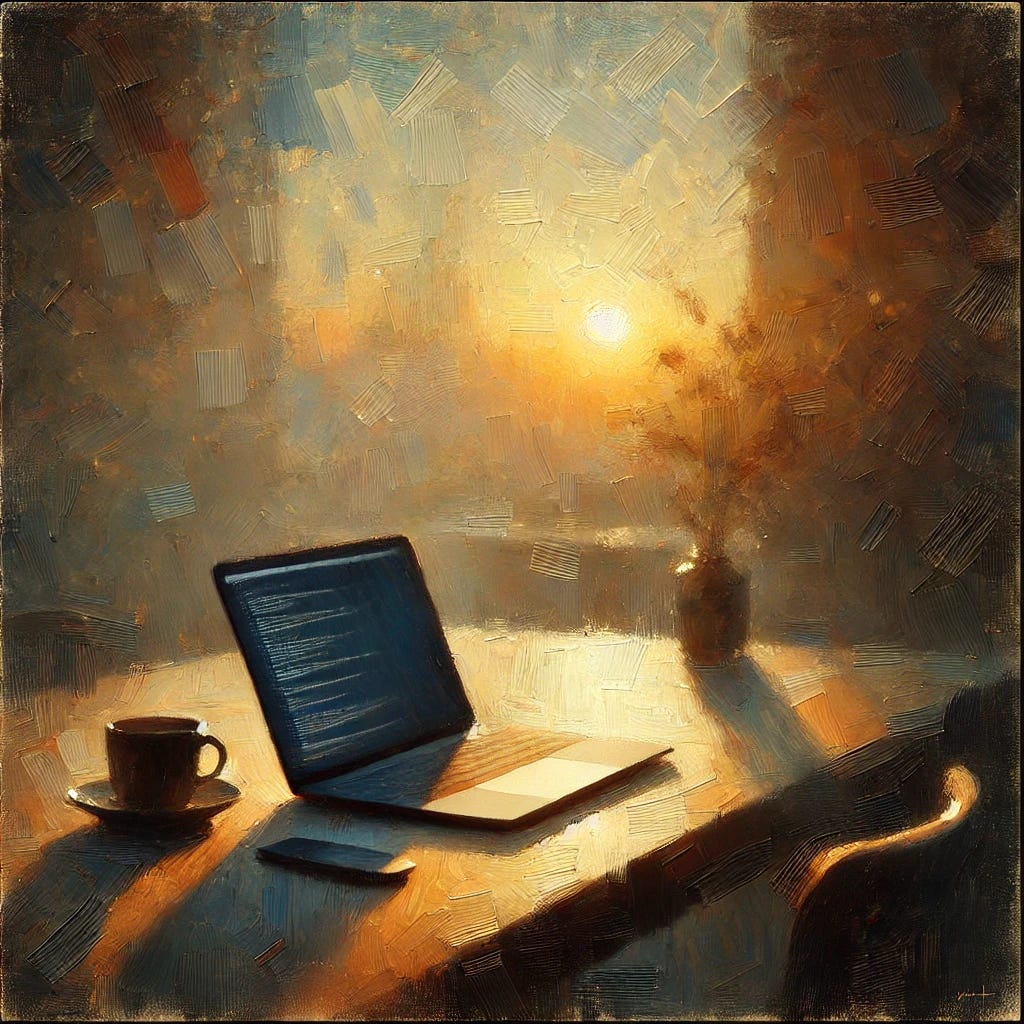
Let’s talk about jQuery — the library that taught JavaScript how to dance, and by “dance,” I mean wrangle the chaos of browsers into something resembling harmony. If JavaScript was the wild west of web development, jQuery was the sheriff. But do you still need this sheriff in a world of modern frameworks? Let’s dig in.
What Is jQuery?
jQuery is a lightweight JavaScript library that simplifies the process of writing JavaScript. Instead of writing pages of code to manipulate the DOM (Document Object Model), make AJAX requests, or handle events, jQuery lets you do all that in just a few lines. Its famous tagline, “Write less, do more,” sums it up perfectly.
Example: Instead of this verbose vanilla JS code:
document.querySelectorAll(".button").forEach(function(el) {
el.addEventListener("click", function() {
alert("Button clicked!");
});
});
You’d write this in jQuery:
$(".button").click(function() {
alert("Button clicked!");
});
Magical, right?
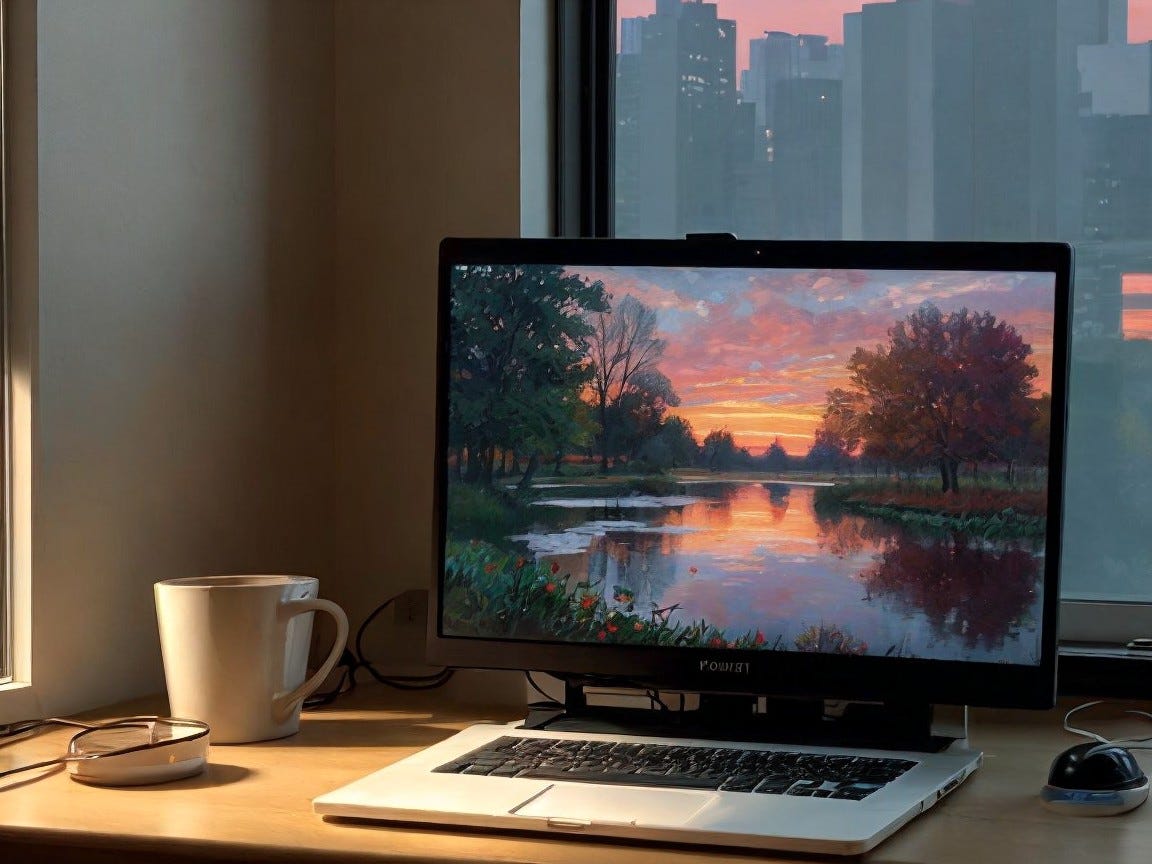
Why Should You Care About jQuery?
Even if you’re fluent in modern frameworks like React, Vue, or Angular, jQuery offers a certain simplicity that’s hard to beat for quick, small projects. It’s also deeply embedded in the DNA of the web, so understanding it can make debugging legacy systems much easier.
Plus, let’s face it — who doesn’t love the efficiency of chaining methods?
Is jQuery Still Relevant?
Short answer: Yes, but it depends.
Long answer: jQuery isn’t winning any “Shiny New Thing” awards these days, but it’s far from obsolete. According to W3Techs, jQuery is still used by over 70% of websites. That’s like being the Beatles of JavaScript libraries.
If you’re maintaining legacy code or working on smaller projects, jQuery might still be your best friend. For modern apps, frameworks like React or Svelte have largely stolen the spotlight.
How Widely Used Is jQuery?
jQuery is so widely used that even websites from the modern age still rely on it. Think of it as the duct tape of web development: not glamorous, but it holds things together. Popular platforms like WordPress ship with jQuery, and a vast ecosystem of plugins has been built around it.
A Brief History of jQuery
John Resig unleashed jQuery onto the world in 2006. Back then, working with JavaScript meant braving a warzone of browser inconsistencies. jQuery came in as the mediator, providing a unified API that worked across browsers. By the late 2000s, jQuery was the tool for web development, loved for its ability to make JavaScript accessible.
Fast forward to today: jQuery is no longer at the bleeding edge, but it remains a stalwart of the web.
Real-Life jQuery Example
Let’s say you’re building a website with a “Back to Top” button. Here’s how you’d implement it with jQuery:
$(document).ready(function() {
$(window).scroll(function() {
if ($(this).scrollTop() > 100) {
$(".back-to-top").fadeIn();
} else {
$(".back-to-top").fadeOut();
}
});
$(".back-to-top").click(function() {
$("html, body").animate({ scrollTop: 0 }, 600);
return false;
});
});
Without jQuery, this would require significantly more code (and probably more aspirin).
Technologies That jQuery Plays Nice With
jQuery is like that easy-going friend who gets along with everyone. You can use it with PHP, Ruby on Rails, ASP.NET, and more. It’s also compatible with front-end tools like Bootstrap (which used to rely on it heavily) and works seamlessly with AJAX for server-side communication.
Famous Projects Using jQuery
While newer projects lean towards modern frameworks, countless big names still have jQuery under the hood. Think WordPress, Tumblr, and Shopify themes. It’s often hidden in the background but still does heavy lifting.
Best References for jQuery
- jQuery Official Documentation
- jQuery Learning Center: Offers tutorials and documentation to help you understand and use jQuery effectively.
- W3Schools jQuery Tutorial: Provides comprehensive tutorials and examples for learning jQuery.
Any Interesting Tidbits?
- Chaining Methods: You can chain almost every method in jQuery, making your code super concise.
- Plugins Galore: jQuery has thousands of plugins. Need a date picker? Carousel? Modal? There’s a plugin for that.
- Browser Compatibility: jQuery made IE6 tolerable. If you never had to deal with that, consider yourself blessed.
AI Art Prompt:
“An impressionist painting of a serene coder’s desk at dusk, lit by soft natural light, with minimalist tools like a single laptop and a coffee cup. No glowing screens or harsh light — just the essence of quiet focus and simplicity.”
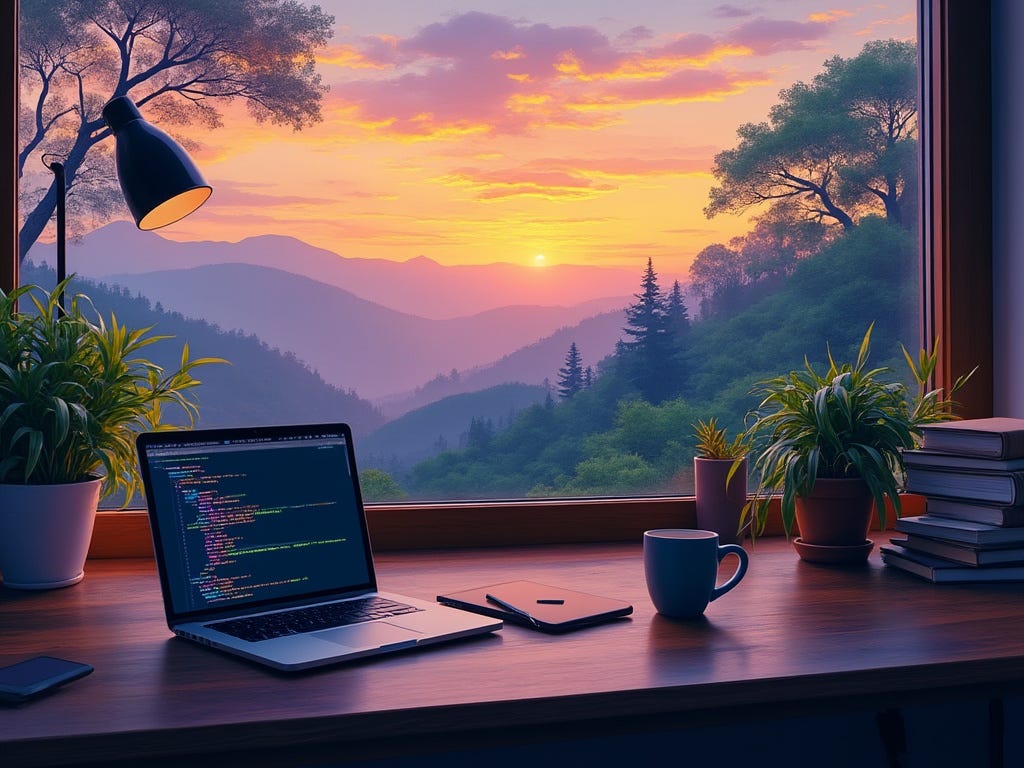
Got thoughts on jQuery? Share them in the comments! Follow for more web dev musings, and let’s keep the conversation going.