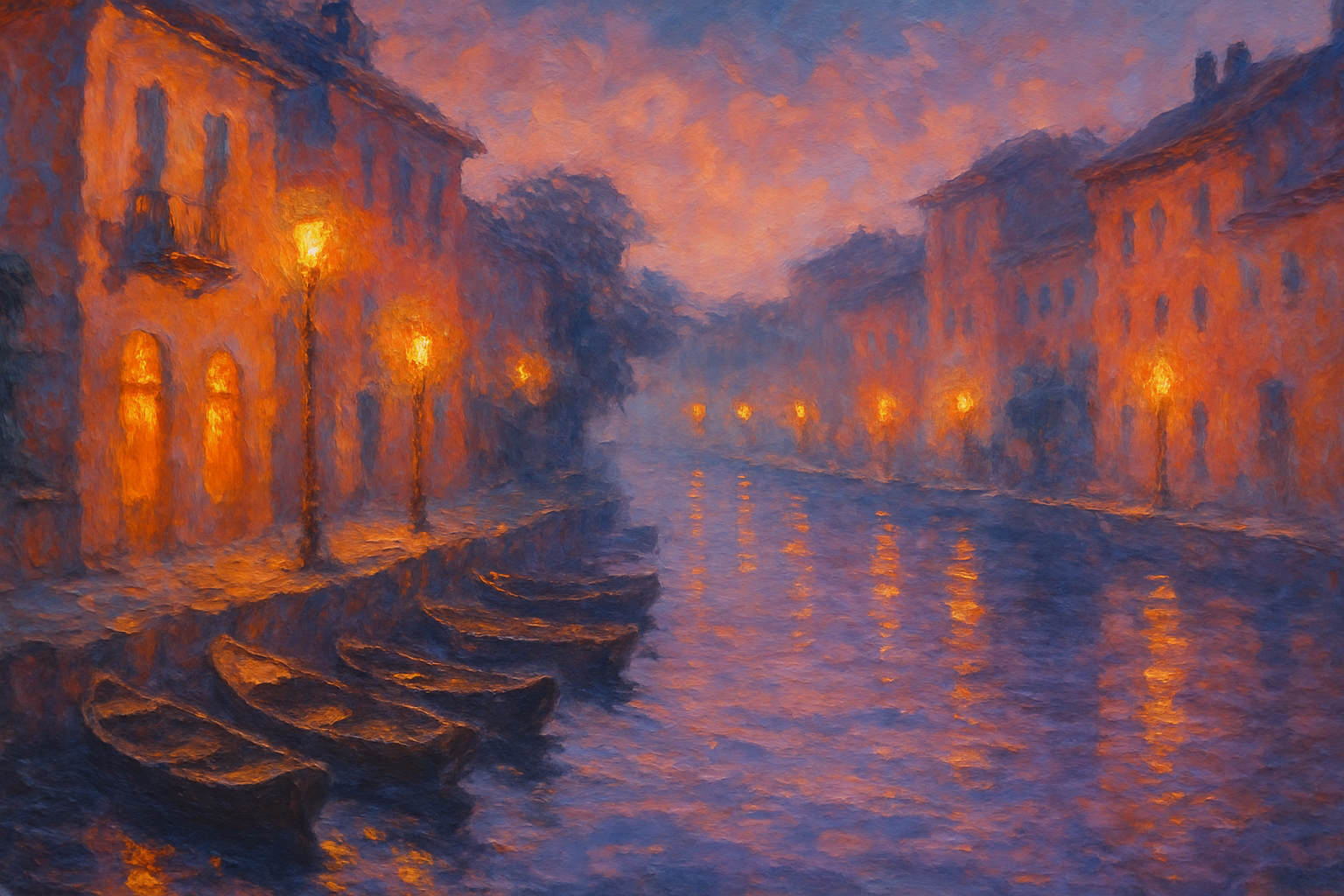
Laravel for Laughing (and Learning)
Welcome, brave developer! You’re here because you heard about Laravel — PHP’s golden child — and thought, “How hard could this be?” Well, buckle up, buttercup. We’re diving into a PHP framework that’s part Swiss Army knife, part magician, and part diva (but the lovable kind that insists on elegance).
Laravel is the Beyoncé of PHP frameworks. It wants your code clean, your syntax sweet, and your web apps gliding like an ice skater on butter. So how do you get started?
Step 0: Prerequisites (Or: Don’t Bring a Spoon to a Framework Fight)
Before you even whisper “Artisan,” you’ll need:
- PHP ≥ 8.1 (Laravel doesn’t do basic)
- Composer (think of it as Laravel’s butler)
- A basic understanding of PHP (but Laravel will let you fake it for a while)
💻 Installing the Goods
macOS (with Homebrew):
Laravel loves macOS users like a hipster loves pour-over coffee:
brew install php composer
Ubuntu/Debian (AKA “The real developer starter pack”):
sudo apt update
sudo apt install php php-cli php-xml php-sqlite3 unzip curl
curl -sS https://getcomposer.org/installer | php
sudo mv composer.phar /usr/local/bin/composer
Windows: Go to getcomposer.org. Laravel won’t judge you. Much.
💡 Running this in a virtual machine? Laravel inside Multipass is smooth as silk on waffles. It just needs a bit of setup, so read that guide if you’re in a VM.
Step 1: Install Laravel (Like Summoning a Friendly Code Demon)
Open up your terminal and cast this glorious incantation:
composer create-project laravel/laravel hello-world-app
Composer will sing the song of its people, download roughly one bazillion dependencies, and leave you with a fresh new folder called hello-world-app
, filled with mysterious treasures like routes
, config
, and something called Artisan
, who is not a French baker but may as well be.
Step 2: Set Up SQLite (Because Databases Deserve Simplicity Too)
You want a database, but you don’t want a relationship. We get it. Enter SQLite, Laravel’s low-maintenance ex.
First, slide into the project directory and create the database file:
cd hello-world-app
mkdir -p database
touch database/database.sqlite
Now tell Laravel, “This is where we keep the feelings” by editing .env
:
DB_CONNECTION=sqlite
DB_DATABASE=/absolute/path/to/hello-world-app/database/database.sqlite
Replace the path with your actual one. Need help?
pwd
Finally, run the migrations to create necessary tables (like sessions
), so Laravel doesn’t throw a tantrum about missing furniture:
php artisan migrate
Step 3: Run It (Cue the Trumpets)
You’re ready to see something in the browser.
🖥️ If you’re on your local machine (like a sensible person):
php artisan serve
Open your browser and head to:
http://127.0.0.1:8000
Boom. You’ve just launched Laravel’s homepage.
🧱 Running inside a VM?
Laravel defaults to only serving localhost
, so this won’t work unless you explicitly tell it to open the front door:
php artisan serve --host=0.0.0.0
Then, from your host machine, visit:
http://<your-vm-ip>:8000
e.g.,
http://192.168.64.5:8000
If that still doesn’t work, make sure port 8000 is open:
sudo ufw allow 8000
And yes, Laravel will now finally talk to you through the firewall.
Step 4: Routing to Your Hello World
Let’s get Laravel to say something other than “Hello, Welcome, Please install Jetstream.”
Open routes/web.php
and replace whatever’s there with this:
use Illuminate\Support\Facades\Route;
Route::get('/', function () {
return 'Hello, World!';
});
Now refresh the browser.
Voilà. Laravel is now saying something custom — something real — something… yours.
Step 5: Let’s Get Fancy with Views
So far we’ve been returning raw strings like a PHP caveman. Let’s class it up with Blade, Laravel’s templating engine (not to be confused with Wesley Snipes).
First, create a view:
resources/views/hello.blade.php
And put this inside:
<!DOCTYPE html>
<html>
<head>
<title>Hello Blade</title>
</head>
<body>
<h1>Hello, World from Blade!</h1>
</body>
</html>
Update your route:
Route::get('/', function () {
return view('hello');
});
Now you’ve got HTML rendering through Laravel. You’re not just coding now — you’re developing.
Step 6: Controller Time (Because You’re All Grown Up)
Now that you’re mature and responsible (and wearing clean socks), you’re ready for a controller.
Make one:
php artisan make:controller HelloController
Laravel will nod approvingly and give you a new file: app/Http/Controllers/HelloController.php
Open it and make it say hello:
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class HelloController extends Controller
{
public function greet()
{
return view('hello');
}
}
Then update your route once more:
use App\Http\Controllers\HelloController;
Route::get('/', [HelloController::class, 'greet']);
You’re now doing MVC. You’re basically a software architect. Start putting “Senior” in your email signature.
What Now?
You’ve built a working Laravel app. It says “Hello.” It serves HTML. It knows how to behave in polite company.
You could stop here… but Laravel’s just warming up. Go deeper:
Final Thoughts
Laravel makes PHP feel like the cool kid again. With great tools, expressive syntax, and a passionate community, it’s got all the charm of Ruby on Rails without the beard oil.
Now go build something ridiculous. Or serious. Or a serious app that’s ridiculous underneath. Laravel doesn’t judge.
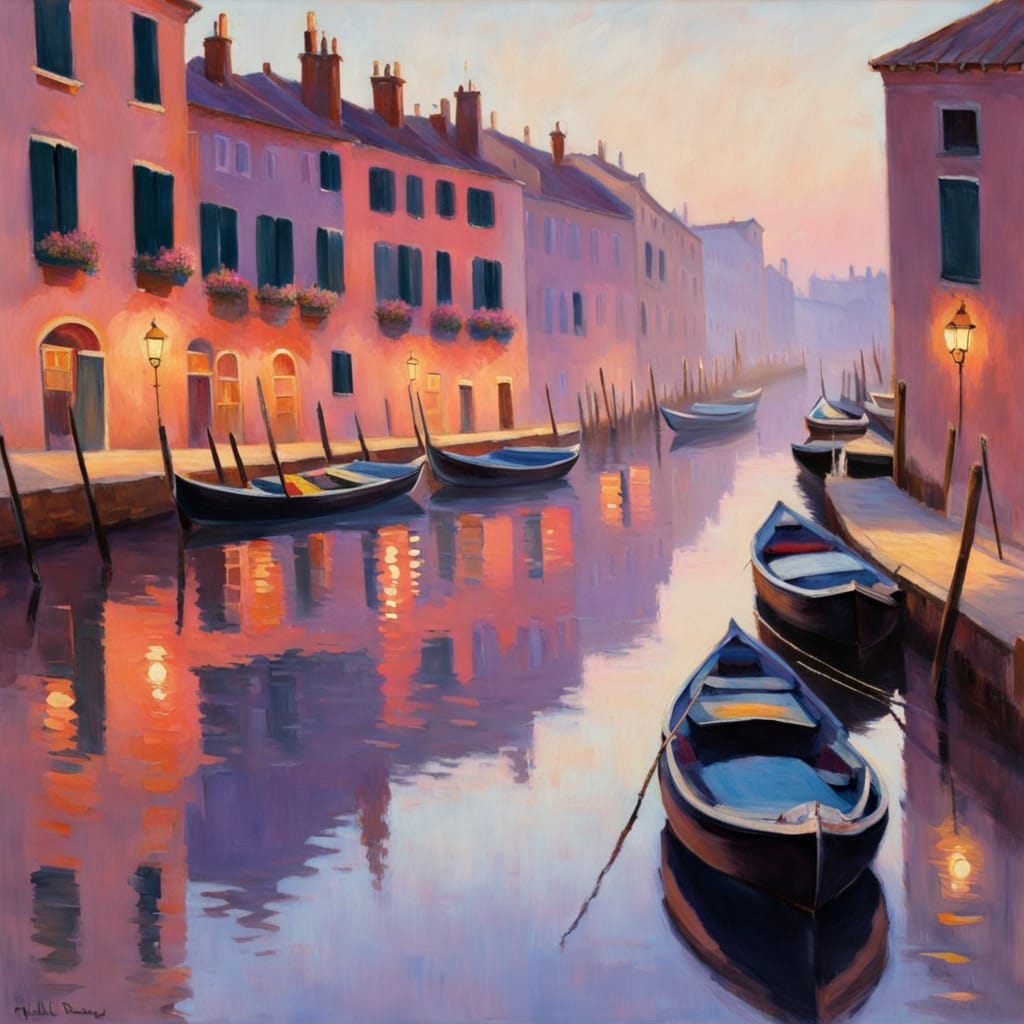
Art Prompt:
A glowing impressionist canal scene at dusk, with lamp-lit reflections rippling through soft lavender water. Rowboats line the banks, their shadows stretching into the mist. The buildings are painted in sunset hues of coral and deep peach, with hints of blue shadow under their balconies. The brushwork is loose and shimmering, creating a dreamy, romantic atmosphere.
Got questions? Got Laravel jokes? Got a bug that’s making you scream into your keyboard? Drop a comment. And don’t forget to follow for more tech tutorials that keep the fun in function.