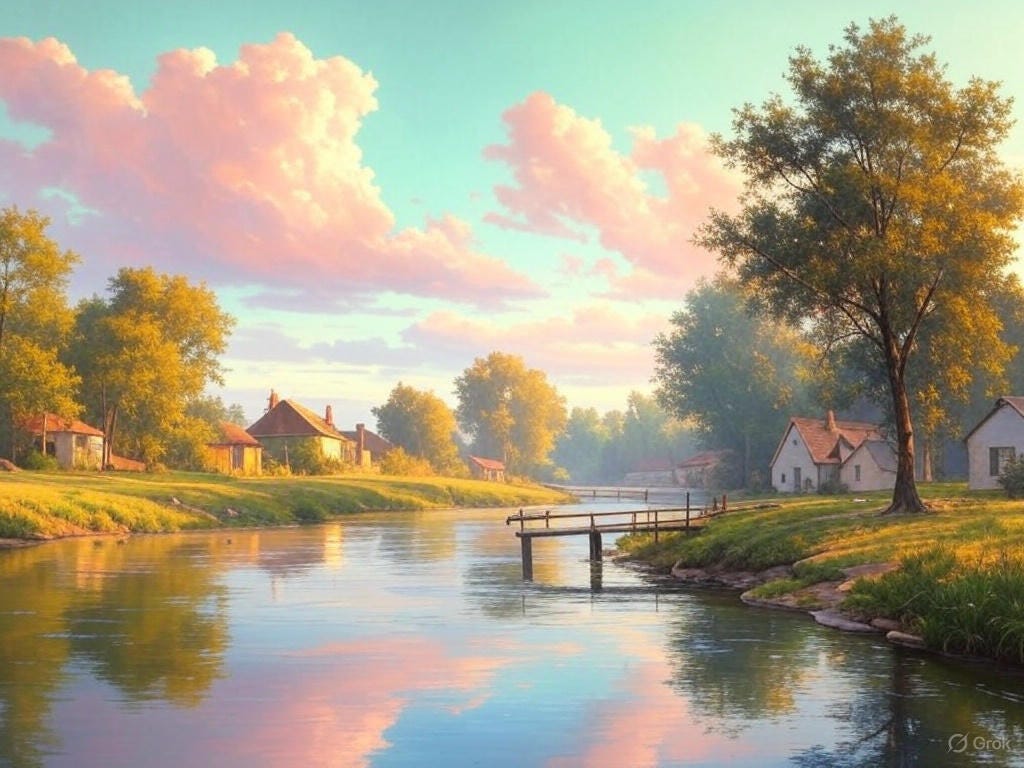
Ah, Flask-RESTful. The duct tape of Python APIs. Elegant as a penguin in a tuxedo, yet scrappy enough to survive in the wild world of web dev. If you’ve ever wanted to build an API without spinning up the Death Star (we’re looking at you, Django REST Framework), then buddy, you’re in the right place.
Let’s break this down like a clumsy IKEA shelf.
What is it?
Flask-RESTful is an extension for Flask that adds support for quickly building REST APIs. It’s like a friendly intern who says, “Hey, I organized your endpoints by resource and added HTTP method support — hope that’s cool.”
Is it still relevant?
Still relevant? Absolutely. It’s the API framework equivalent of that one hoodie you’ve had for ten years — it still works, it still fits, and somehow, it hasn’t caught fire. It’s not the shiny new hotness, but it is rock-solid and low-drama.
Pros and Cons
Pros:
- Super lightweight and flexible.
- Easy to learn — great for beginners and pros who like to freestyle.
- Integrates naturally with Flask.
- Extensible with minimal fuss.
- Very readable code — doesn’t feel like wrestling with a dishwasher manual in Klingon.
Cons:
- Doesn’t include all the bells and whistles like authentication, rate limiting, or built-in schema validation.
- Less opinionated, which means more decisions for you. Like, all of them.
- Not ideal for very large projects (unless you like chaos and stack traces that haunt your dreams).
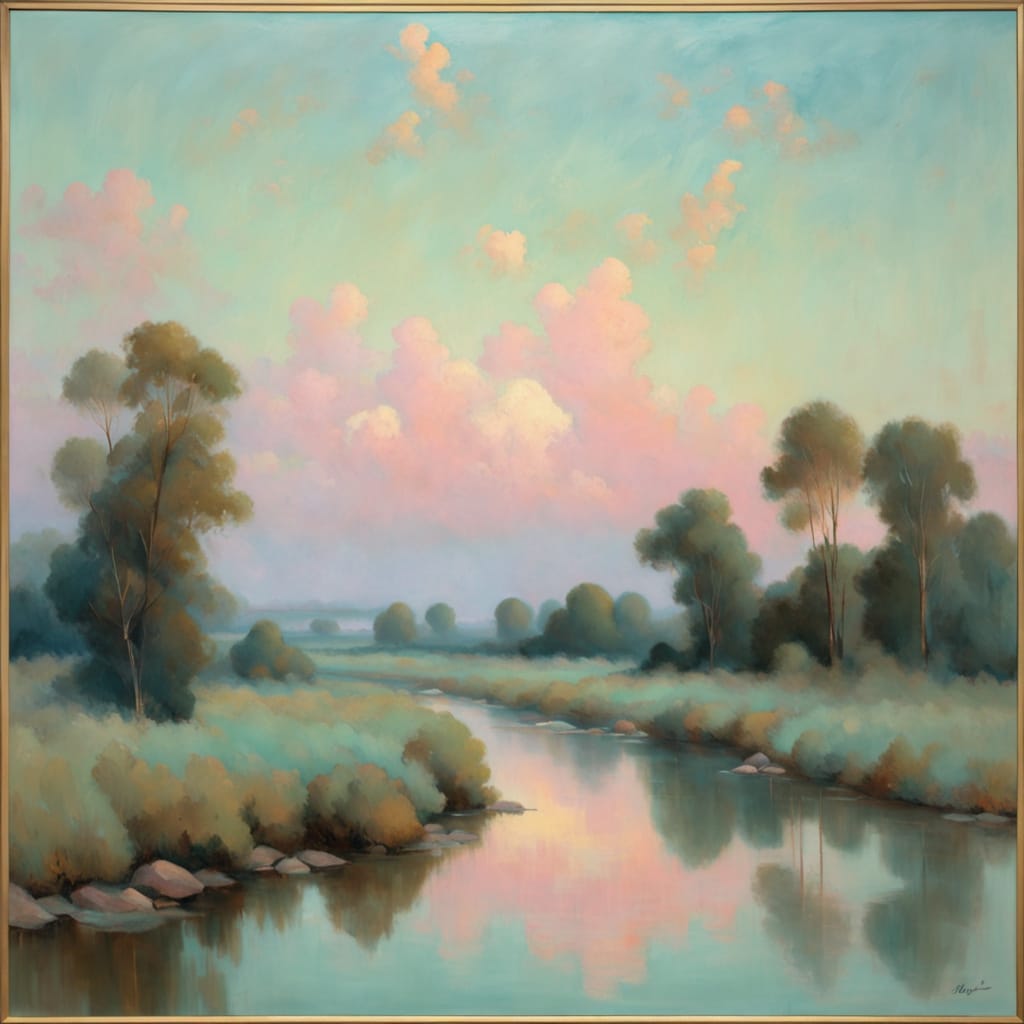
Strengths and Weaknesses
Strengths: Speed of development, simplicity, customizability. You’re the boss here.
Weaknesses: You’re also the IT department, security auditor, and architect. Good luck.
What is it used for?
It’s mostly used to build RESTful APIs. Think JSON in, JSON out. It’s perfect for microservices, internal tools, prototypes, or that weird side project you swear you’ll finish this time.
Can you give me an example?
Of course, here’s how you create an API that says hello. It’s friendlier than most of your relatives at Thanksgiving.
from flask import Flask
from flask_restful import Resource, Api
app = Flask(__name__)
api = Api(app)
class HelloWorld(Resource):
def get(self):
return {'hello': 'world'}
api.add_resource(HelloWorld, '/')
if __name__ == '__main__':
app.run(debug=True)
Boom. You just made an API endpoint in 10 lines. Somewhere, a Django developer is weeping.
Alternatives?
Oh, there are plenty. Buckle up.
- FastAPI: Fast, modern, includes automatic docs via OpenAPI. Like Flask-RESTful’s cooler, trendier cousin with a startup job and a protein shake.
- Django REST Framework: Big, powerful, enterprise-ready. Also, complex.
- Tornado: If you need asynchronous support and enjoy danger.
- Falcon: Lightweight, performant, and very strict. Basically the opposite of Python’s “we’re all consenting adults” motto.
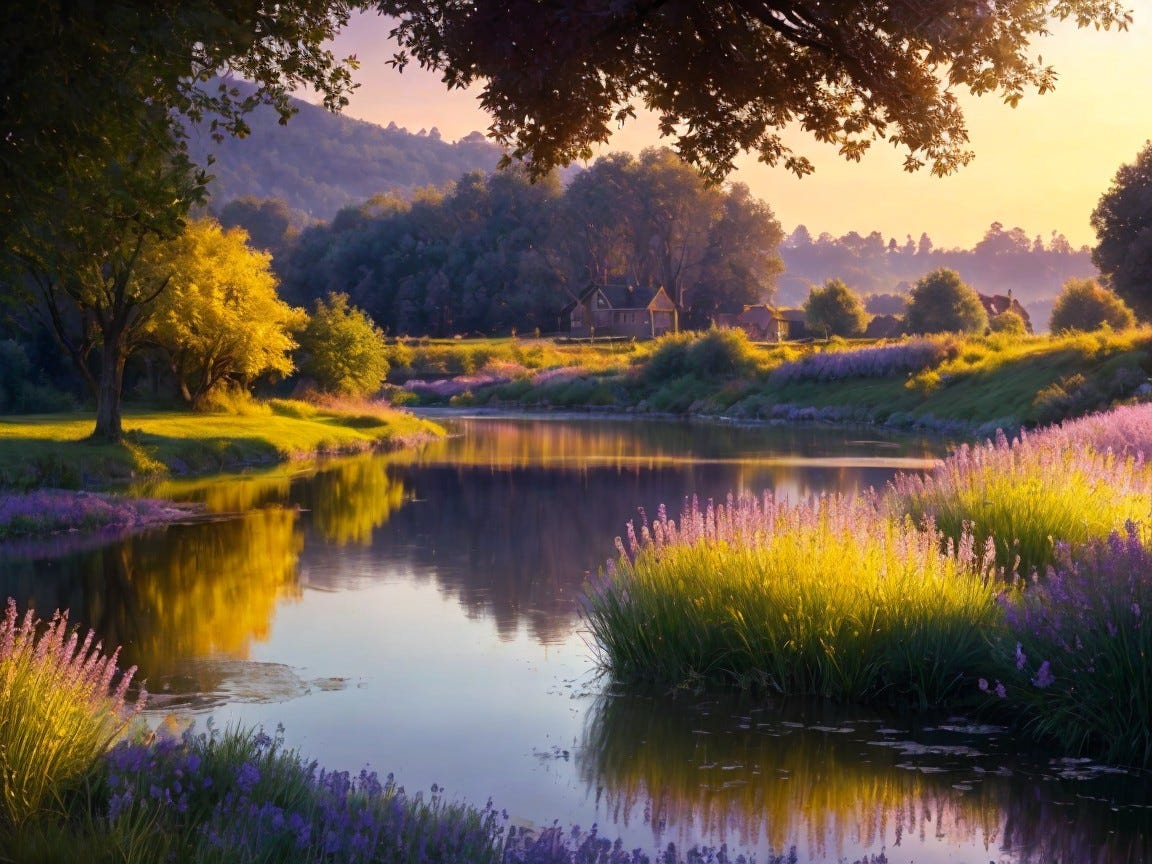
Is it the subject of any famous art?
You jest, but honestly, I imagine Monet would’ve painted it if he’d been into code instead of lilies. It’s got that minimalism he loved. Plus, Flask-RESTful is the digital version of a serene pond: calm on the surface, custom logic raging underneath.
How popular is it?
Still solidly popular, especially in educational settings, smaller startups, and hobbyist projects. It’s like that indie band that never sold out but still gets booked every summer.
Is it going up or down in popularity?
It’s holding steady. Not a meteoric rise, but not crashing either. Think: dependable Subaru. FastAPI is gaining traction fast, but Flask-RESTful isn’t sweating it.
When was it most popular?
Around the 2015–2019 era, before FastAPI came swaggering onto the scene like a TypeScript-fueled cowboy. But Flask-RESTful is still actively maintained and used.
What is its history?
It grew out of the Flask community’s desire for a simpler way to build REST APIs. Flask itself is micro, and RESTful piggybacked on that minimalism to become the cool REST kid on the block.
Who invented it?
Flask-RESTful was originally developed by Michael Gerstenhaber, a Twilio engineer, to make building REST APIs with Flask more structured and pleasant. It later became part of the broader Flask ecosystem and is now community-maintained by kind-hearted Pythonistas who love lightweight tools.
What companies use it the most?
Startups, internal tools teams, and any dev who says “meh, I’ll just spin up a quick API” and then six months later has a 47-route monster humming along in production.
Is it similar to anything else?
Yes — FastAPI, Django REST Framework, Falcon, and even Express.js (if you squint really hard and ignore the JavaScript). All are trying to do what Flask-RESTful does: expose logic over HTTP. Flask-RESTful just prefers to do it in sweatpants.
Does it work well with AI?
Absolutely. Build your own AI inference endpoints, model monitoring tools, or chat API middlemen. It plays nicely with TensorFlow, PyTorch, OpenAI APIs, huggingface, and the whole alphabet soup.
What tech stack does it work with?
Flask-RESTful runs in any Python 3.x environment. Typically paired with:
- SQLAlchemy for ORM.
- Marshmallow or Pydantic for validation (though you roll your own usually).
- Gunicorn or uWSGI for deployment.
- Docker for shipping it like a professional.
What tools work best with it?
- Postman — for testing your endpoints.
- Flask-SQLAlchemy — if you’re feeling relational.
- Marshmallow — for object serialization/deserialization.
- Swagger UI — with some extra config.
Any other interesting tidbits?
- You can totally use class-based views, which is rare in microframework land.
- You can define custom HTTP response codes with a flick of your pinky.
- Its error handling system is surprisingly elegant.
- Great for teaching REST concepts to new devs. It’s like giving someone a lightsaber that can’t accidentally slice off a limb.
So, want more smart nonsense like this? Follow for more dev musings, frameworks roasts, and occasional API appreciation poetry. Comment below if you’ve used Flask-RESTful and survived, or if you have hot takes about why FastAPI is better (you’re wrong, but it’s okay).
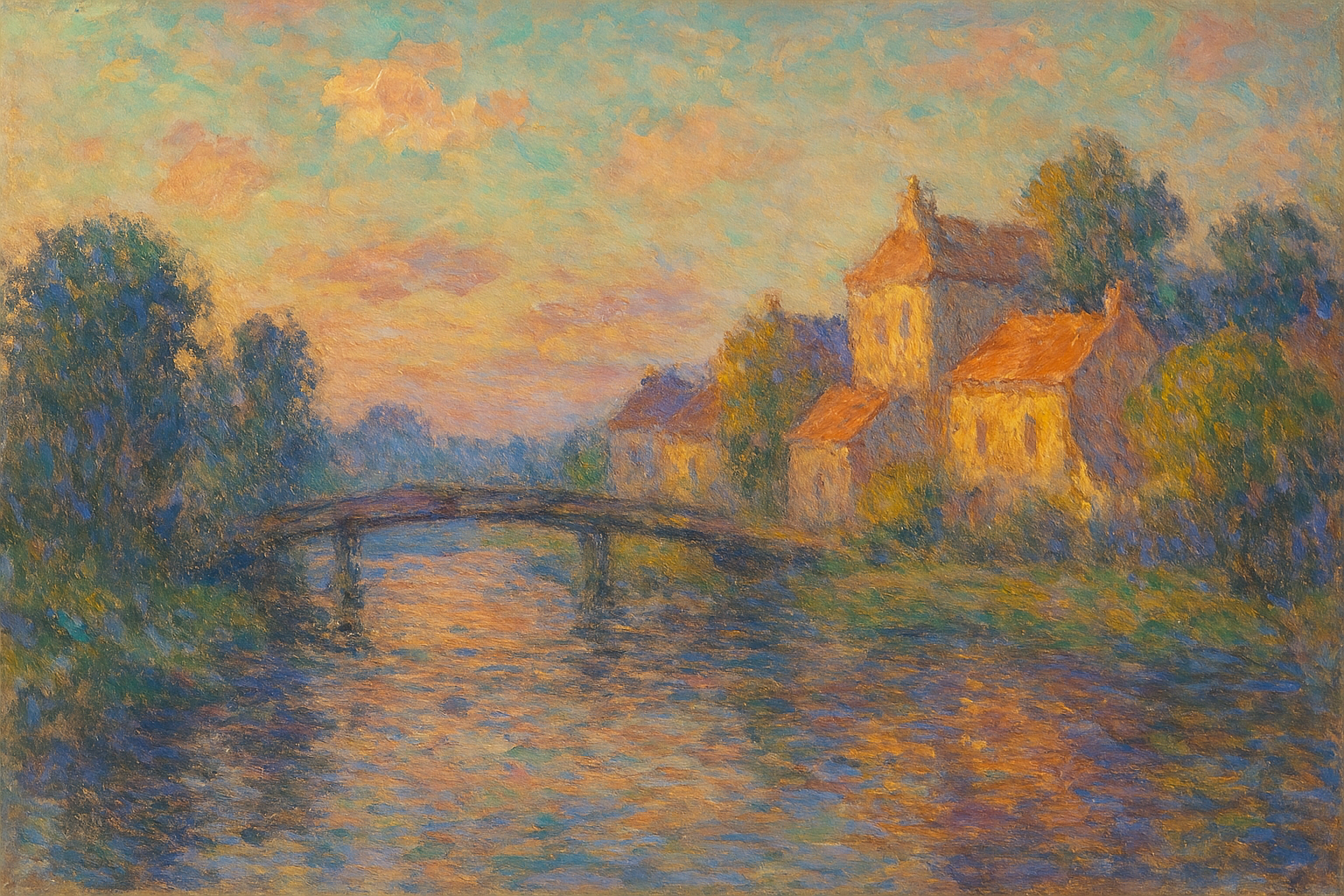
Art Prompt:
A tranquil landscape at dusk, painted with soft, sweeping brush strokes like those used by 19th-century Impressionist masters. The scene depicts a modest, sunlit village at the edge of a riverbank, with shimmering reflections on the water that blend warm golds and cool lavenders. A narrow footbridge arches gently across the river, and the trees are rendered with visible dabs and dashes of green, blue, and ochre. The sky is expansive, dotted with strokes of rosy pink clouds drifting across a pale turquoise backdrop. The entire painting has a dreamlike haze, evoking a sense of fleeting beauty and serene transience.