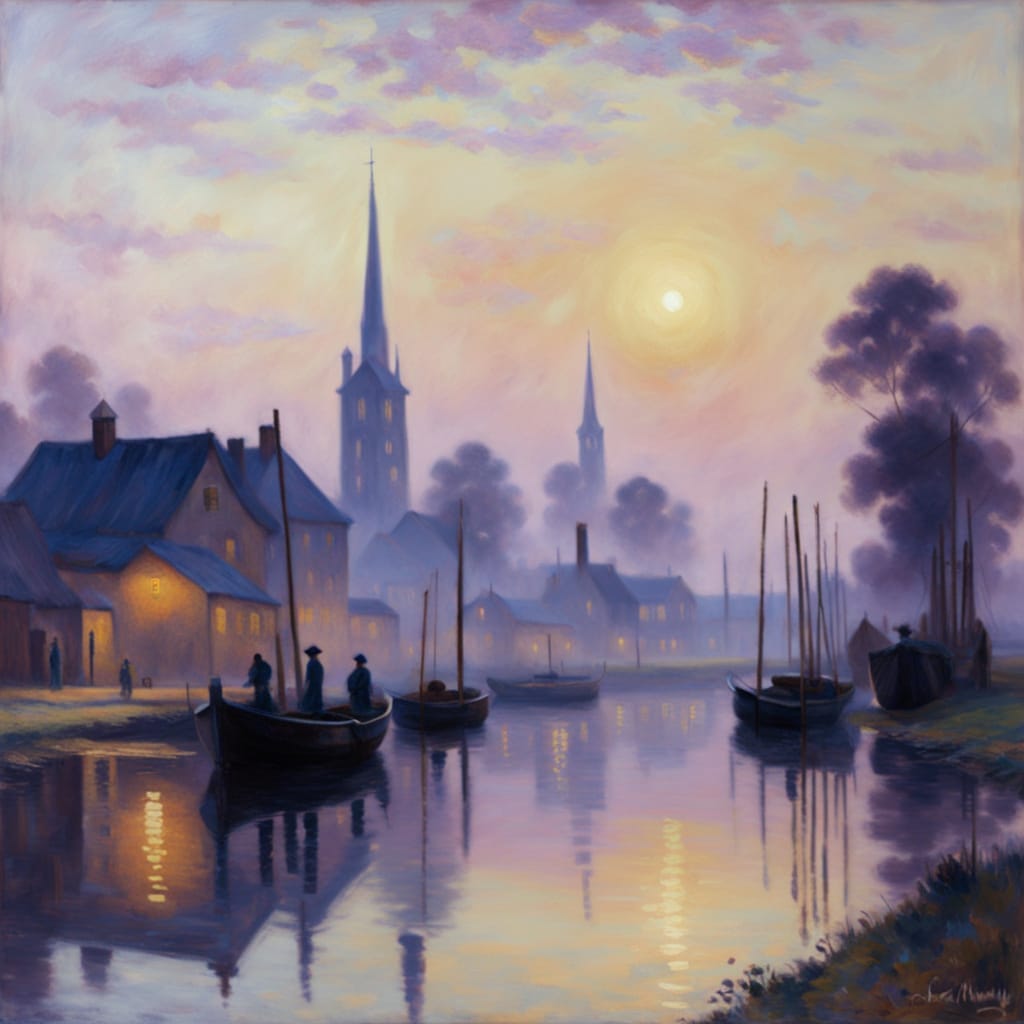
Let’s talk about DataLoader — the silent intern of your AI pipeline who does 95% of the work and still gets no credit. This is the part of the workflow that’s like the roadie at a rock concert. Without it, the lead guitarist (your model) is just a sweaty guy holding a plank of wood on a silent stage.
What is it?
At its core, DataLoader
is a class in PyTorch that lets you iterate over your dataset like a civilized being instead of whatever nonsense you were doing before (probably a bunch of for-loops and regrets). It’s like turning your massive pile of training data into digestible little spoonfuls of data pudding.
from torch.utils.data import DataLoader
loader = DataLoader(my_dataset, batch_size=32, shuffle=True)
Boom. Now your data is sliced, diced, shuffled, and served to your model like a Michelin-starred buffet line.
Is it still relevant?
Is oxygen still relevant? Yeah. It is. So is DataLoader
. Unless you’ve moved to some alien dimension where data magically feeds itself into models, you’re gonna need a DataLoader (or at least something like it).
Pros and Cons
Pros:
- Batching, shuffling, and multiprocessing — all in one.
- Works with any custom dataset you build.
- Makes you look like you know what you’re doing.
Cons:
- Can get a bit clunky with weird data formats.
- Multiprocessing issues on Windows. (Yeah, Windows, it’s always you.)

Strengths and Weaknesses
Strengths: Parallel loading, customizable collate functions, and an uncanny ability to make your training faster without changing your code much.
Weaknesses: You need to understand how datasets work first. Otherwise, you’ll stare at __getitem__
like it just insulted your ancestors.
What is it used for?
Feeding data to your model. It’s like a conveyor belt at a sushi restaurant. Without it, you’d just have one giant tuna lying on your table. Good luck training on that.
Can you give me an example?
Sure. Say you’re building a cat vs dog classifier (very original, wow). You have a folder of 5,000 images of cats and 5,000 images of dogs. DataLoader
helps you:
- Load the images in batches of 64
- Shuffle them so it’s not 5,000 cats followed by 5,000 dogs (bias alert)
- Transform them (resize, normalize, make ’em spicy)
- Feed them to the model while using multiple CPU cores
from torchvision import datasets, transforms
from torch.utils.data import DataLoader
transform = transforms.Compose([
transforms.Resize((128, 128)),
transforms.ToTensor(),
])
dataset = datasets.ImageFolder(root='data/cats_vs_dogs', transform=transform)
loader = DataLoader(dataset, batch_size=64, shuffle=True, num_workers=4)
You’re welcome.
What are the alternatives?
- TensorFlow’s
tf.data.Dataset
: Also excellent, but it’s TensorFlow-flavored. So if you’re in that ecosystem, go for it. - Hugging Face Datasets: Amazing for NLP tasks, handles giant datasets like a champ.
- Custom loaders with
multiprocessing
: If you hate happiness and love debugging.
Is it the subject of any famous art?
Only in spirit. Imagine a beautiful painting of an assembly line full of little data packets being carried by tiny robotic butlers. That’s DataLoader
. Sadly, no one has painted this yet because the art world still doesn’t respect PyTorch. Rude.
How popular is it?
Very. If PyTorch is the band, DataLoader
is the drummer. Not the flashy lead, but without it, everything falls apart and the whole set crashes into a burning heap of static and shame.
Is it going up or down in popularity?
Steady and strong. It’s a built-in part of PyTorch and will probably continue to evolve. Unless some AI godchild invents a better way to move data around (like telepathy), it’s here to stay.
When was it most popular?
Right around the time deep learning went mainstream — 2017 onward. Basically, it’s been riding the AI hype wave like a majestic dolphin.
What is its history?
Born out of PyTorch’s need to handle data efficiently and in parallel. Before DataLoader
, people were manually handling data loading with Python lists and prayer.
Who invented it?
The PyTorch team, aka the same folks who made your model training not feel like medieval torture. Shoutout to the wizards at Meta AI.
What companies use it the most?
- Meta (obviously)
- Google (yes, even they use PyTorch sometimes)
- NVIDIA
- Every startup trying to build the next AI overlord
Is it similar to anything else?
Yes. It’s like a waiter that only brings batches of exactly 32 sushi rolls, pre-cut and shuffled, and does this without you ever having to yell across the kitchen. You tip it in CPU cycles.
Does it work well with AI?
Absolutely. It was literally made for it. It’s not just compatible — it’s soulmates with neural networks.
What tech stack does it work with?
- PyTorch (obviously)
- Python 3.x
- Works on Linux, macOS, and Windows (with occasional grumbling)
- Supports integration with CUDA for GPU training
What tools work best with it?
torchvision.datasets
for imagestorchtext
for text (though that’s a bit of a rabbit hole)albumentations
for cool data augmentationTensorBoard
to see how fast your data is flying into the model
Any other interesting tidbits?
- You can write a custom collate function to do magic (like padding variable-length sequences).
- You can use
pin_memory=True
to speed up GPU transfers. - Want infinite data? Set
drop_last=True
and pretend the leftovers never existed.
Final Thoughts
Is DataLoader
glamorous? No. Is it necessary? Like water, my friend. If you’re training a model and not using a DataLoader
, go sit in the corner and think about your life choices.
Got thoughts? Weird DataLoader
hacks? Existential dread over your collate function?
Drop a comment! And hey — follow me if you want more spicy deep learning takes, humor, and the occasional cat joke.
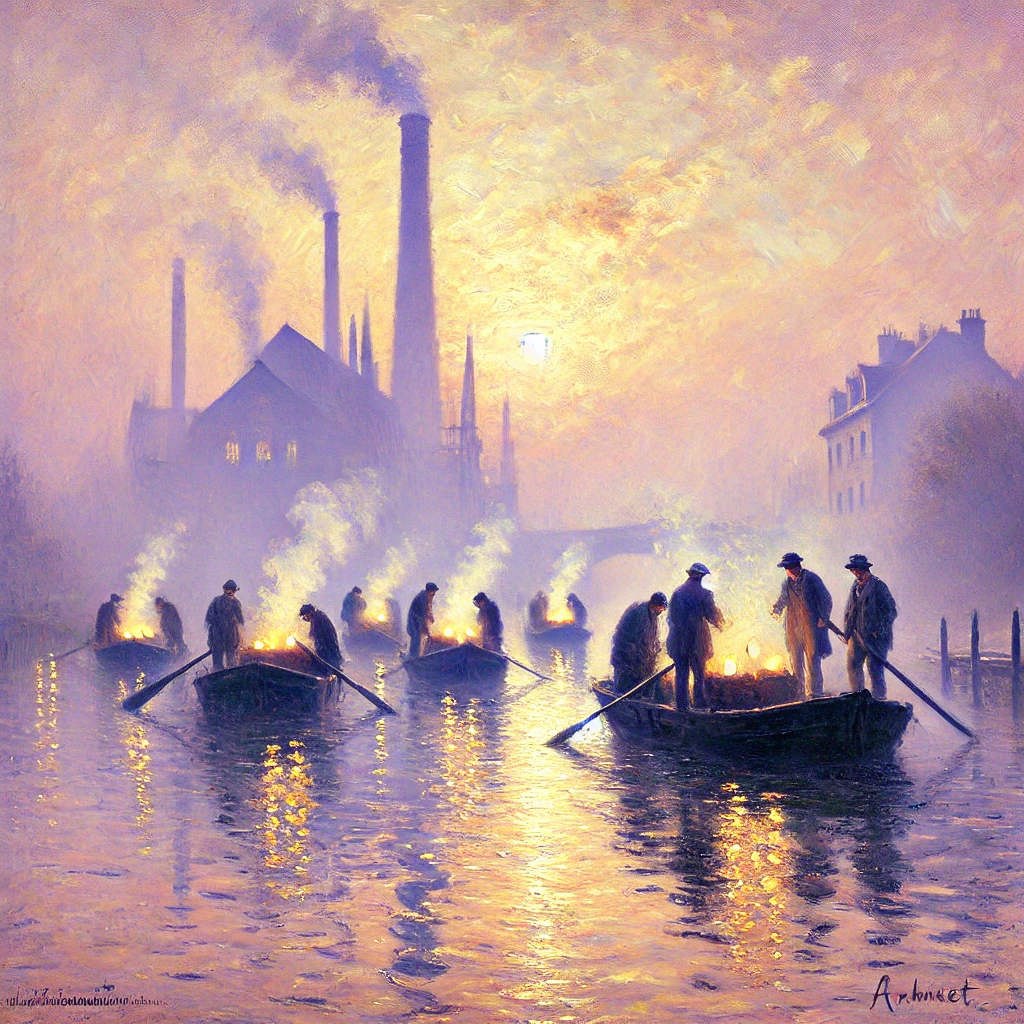
Art Prompt:
A misty riverside village at dawn, rendered in soft pastel tones with impressionistic brush strokes like Monet, where workers in 19th-century garb carry floating glowing orbs from wooden boats into a glowing factory, the entire scene bathed in a pale gold sunrise, water reflections rippling with subtle texture, distant church spire silhouetted against a lavender sky.