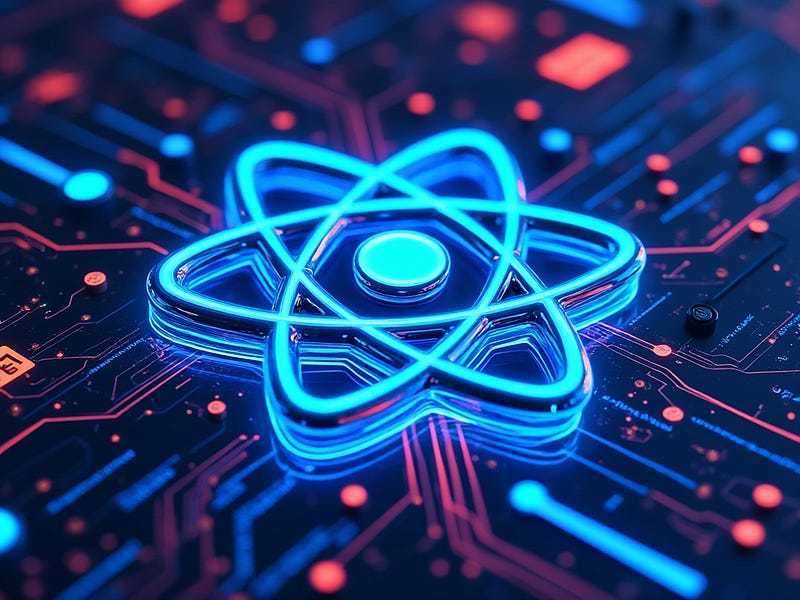
Planning: Sketch It Out First
Start with the bigger picture. Before writing a line of code, clarify your app’s structure, user flow, and data needs. Think components, data flow, and lifecycle hooks. Agile methodologies are ideal for React since they allow rapid iteration and adjustment. Use a project management tool like Jira or Trello to track features, bugs, and improvements and organize your workflow.
Key Resources for Planning:
- React Official Docs: Check out the hooks section and component lifecycle guidelines.
- Trello or Jira: Great for tracking agile sprints and organizing feature development.
Designing Components: Keep It Clean and Modular
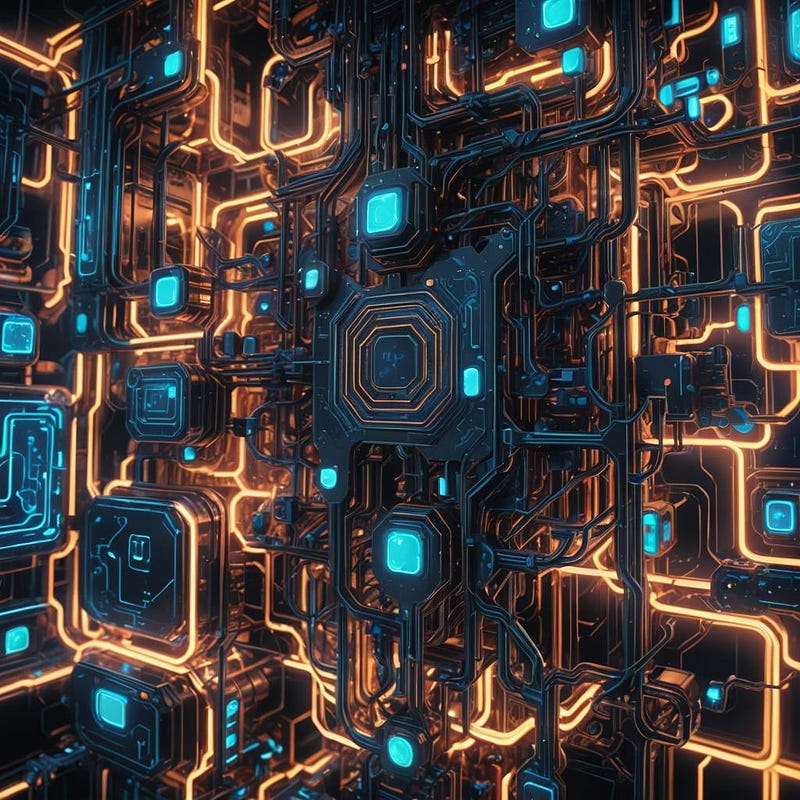
React loves reusable components! Stick to the “Single Responsibility Principle” (SRP) for components — each should do one thing well. Need a button? Create a button component. Need to render a list? Use a reusable list component. Start by outlining a component tree to visualize structure and keep components small and reusable.
- Component Reusability: If you find yourself duplicating code, create a separate component. Embrace functional components, which are lean and easy to test.
- Folder Structure: Organize by function rather than by type. For example, group related components, tests, and assets together. Popular structures include
components
,hooks
,contexts
, andpages
folders.
Design Resources:
- Atomic Design: Great for building complex React UIs with reusable components.
- Storybook: A fantastic tool for documenting and testing UI components in isolation.
Implementation: Keep Code Legible and Scalable
In implementation, focus on clear, maintainable code.
- Use Hooks Over Class Components: Hooks offer concise syntax and avoid common pitfalls with lifecycle methods in class components. Custom hooks can be powerful for reusable stateful logic.
- Props vs. Context API: Use props to pass data to immediate children, but for data shared across the app, like themes or user data, leverage React’s Context API. Use context sparingly, though, as it can bloat and complicate the app if overused.
- Error Boundaries: React lacks a try/catch block for render methods. Use Error Boundaries to handle errors in the component tree and improve UX by showing fallback content instead of a blank screen.
Key Resources for Implementation:
- React Docs on Hooks: An excellent guide to understanding and using hooks.
- Airbnb’s React/JSX Style Guide: Highly recommended for writing clean, consistent React code.
Security: Keep Data Safe and Secure
Security is crucial for any production app. React has built-in protections against basic XSS attacks, but there are extra measures you should take:
- Sanitize Inputs: Don’t trust user inputs. Sanitize them to prevent XSS attacks using libraries like DOMPurify.
- Environment Variables: Avoid exposing sensitive keys (like API keys) in your React code. Use
.env
files to store secrets securely. - HTTPS: Use HTTPS to encrypt data between clients and servers. Services like Cloudflare make it easy to enforce HTTPS.
Security Resources:
- DOMPurify: A powerful tool to sanitize HTML for XSS protection.
- React Security Cheat Sheet: Practical security tips for React apps.
Testing: Cover Your Bases
Testing ensures your app works as expected and helps prevent bugs from cropping up as the app evolves.
- Unit Testing: Use Jest and React Testing Library for unit tests. They’re designed to test components in isolation, ensuring individual units perform correctly.
- End-to-End Testing: For testing workflows and user interactions, go with Cypress or Playwright. These tools can simulate user behavior and interactions, helping ensure your app behaves as intended.
Testing Resources:
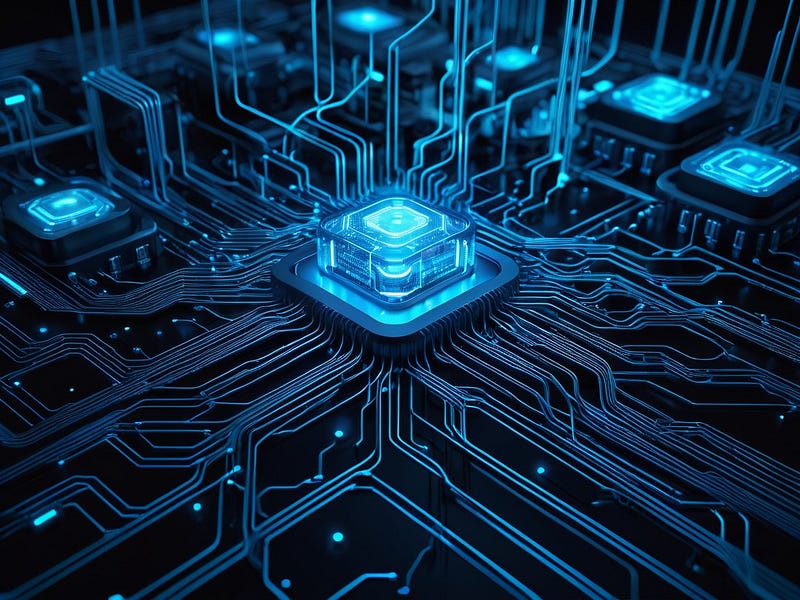
- React Testing Library: Focuses on testing component behavior, not implementation details.
- Cypress: Perfect for e2e tests with a focus on reliability and speed.
Popular Additions to React
Certain libraries and tools complement React nicely, offering features to streamline development or enhance UX.
- React Router: Essential for handling routing in single-page applications (SPAs).
- Redux or Zustand: Redux is great for global state management but consider Zustand for a simpler, lighter approach if Redux feels like overkill.
- Axios or React Query: For making API calls. React Query is especially powerful for data fetching, caching, and synchronization.
Resource:
- React Router Docs: Learn to build navigable and manageable routes.
Databases: Choosing What Works with React
React itself isn’t opinionated about databases, but certain databases work well with it:
- Firebase: Ideal for real-time apps and offers powerful authentication and analytics features.
- MongoDB with Mongoose: Popular for full-stack MERN (MongoDB, Express, React, Node) setups. It’s schema-less, allowing flexibility.
- PostgreSQL with Prisma: For structured data and complex queries, PostgreSQL with Prisma ORM is a solid choice.
Database Resources:
- Firebase: Great for rapid prototyping and real-time capabilities.
- Prisma: A modern ORM that works well with SQL databases.
Open Source Projects for Inspiration
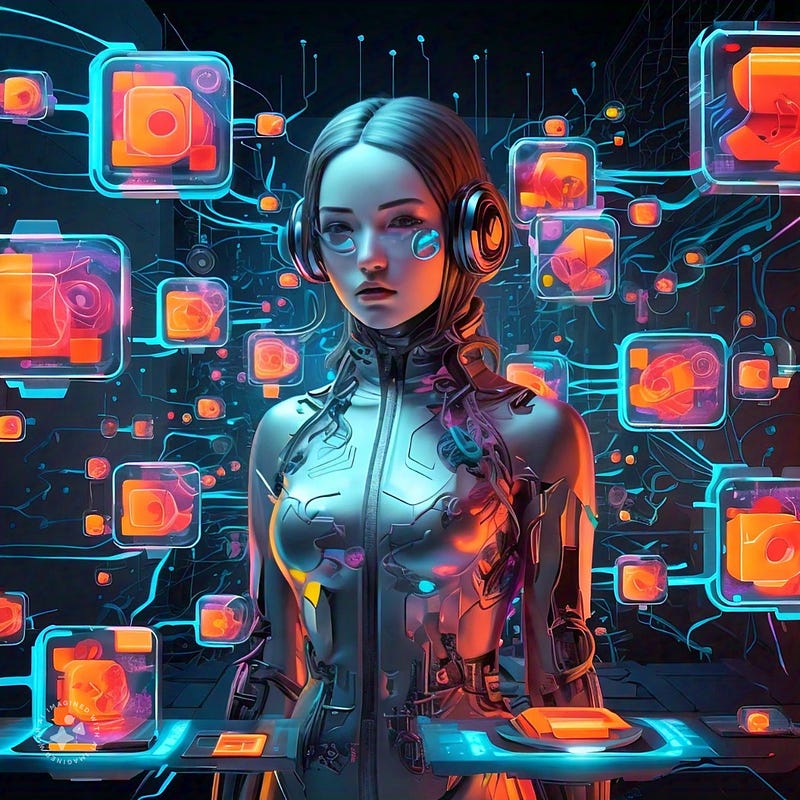
The React community has produced some stellar open-source projects. Here are a few for learning best practices and getting inspired:
- React Admin: A mature React framework for building data-driven admin interfaces.
- Next.js: Even though it’s more than a React library, Next.js shows how to structure a React app for performance and SEO.
AI Tools to Make React Development Easier
AI tools are here to streamline development:
- GitHub Copilot: Assists with coding and automates repetitive tasks.
- Codeium: Provides code completions and can help write tests or refactor code.
- ChatGPT for debugging and prompt generation: Generate code snippets, debug errors, and brainstorm component designs.
AI Art Prompt
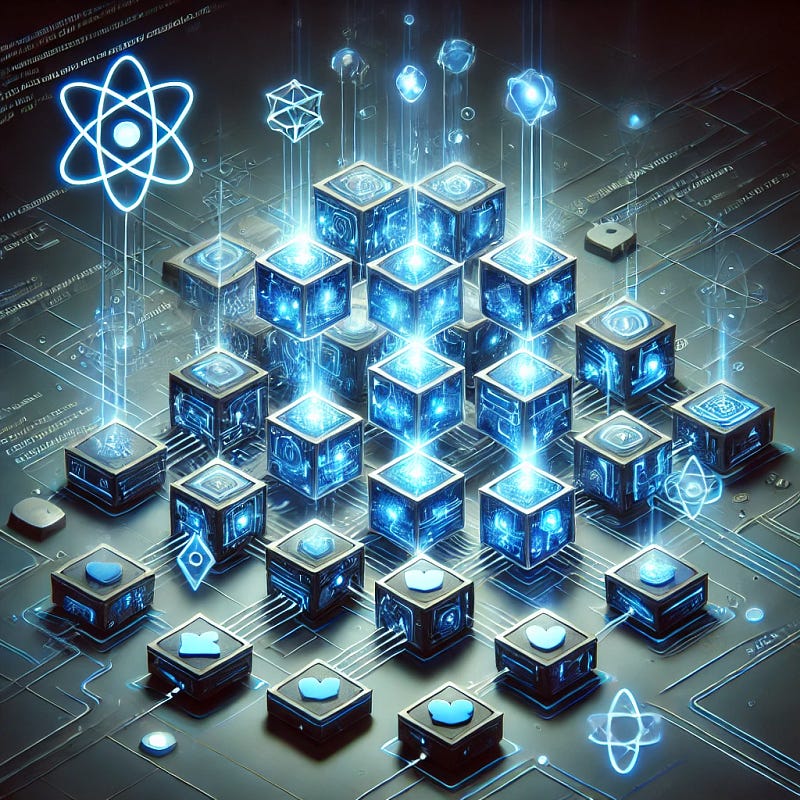
Create a surreal digital painting showcasing a vibrant, interconnected network of React components in a clean and futuristic user interface. Each component is styled like glowing, glassy blocks connected by intricate neon-blue circuits, symbolizing seamless data flow. The background should be a sleek blend of dark and cool hues, with subtle hints of circuitry and code in the design, reflecting the complexity and elegance of a well-organized React application.