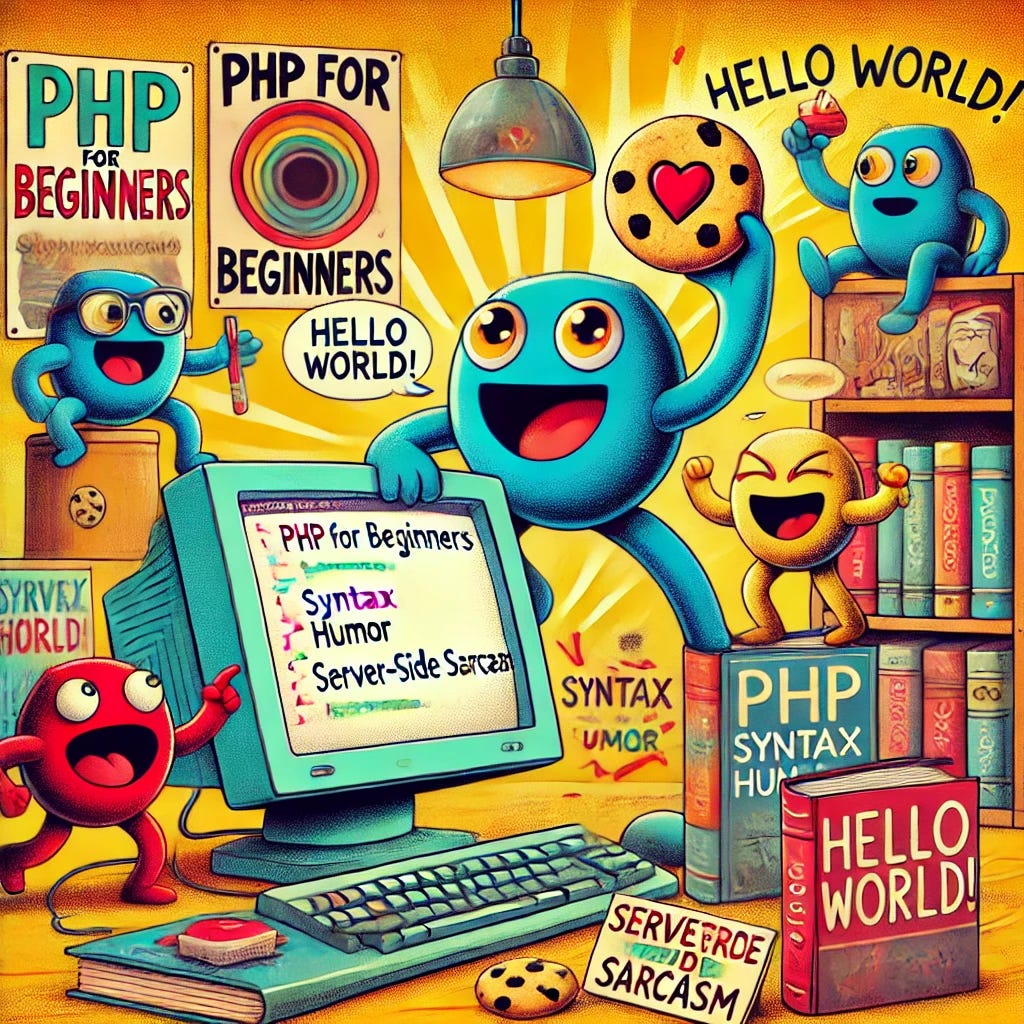
Well, here we are, about to embark on a not-so-serious but terribly important journey into the world of PHP. Why PHP, you ask? Because it’s like the Swiss Army knife of server-side scripting languages — versatile, sometimes mysterious, and always there when you least expect it.
What Even Is PHP?
PHP stands for Hypertext Preprocessor, which is as recursive as a hipster’s playlist. It’s a language designed to make web development feel less like rocket science and more like assembling flat-pack furniture — frustrating at times, but rewarding when you finally get it right.
Why Learn PHP?
- It’s Everywhere: Like that one friend who’s always at every party, PHP is on over 78% of websites.
- Easy to Learn: PHP is like the training wheels of coding. It’s forgiving, friendly, and doesn’t judge you for your semicolon mistakes (too much).
- Community: If you think you’re alone in your PHP journey, think again. There’s a whole community out there, and they’re nicer than your average video game chat.
Getting Started with PHP
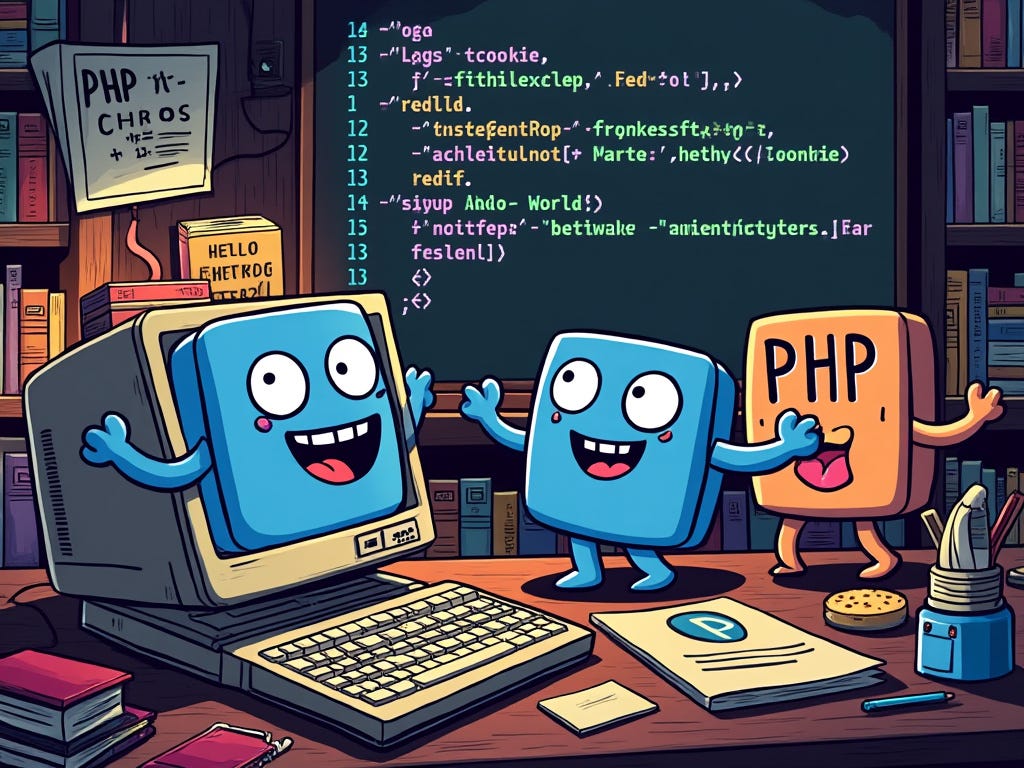
Installation
First things first, you need to set up PHP on your machine. Here are your options:
- Pre-packaged Solutions: XAMPP or MAMP if you’re on a Mac. They come with everything — PHP, MySQL, Apache, and even a free cookie (I made that up).
- Manual Install: Brave souls can download PHP from php.net. Remember, with great power comes great responsibility… and configuration headaches.
Your First PHP Script
Let’s write something simple:
<?php
echo "Hello, World! I'm a PHP script!";
?>
Save this as index.php
in your server’s root directory, and if the stars align and your server isn’t plotting against you, visiting localhost
in your browser should greet you with your message.
PHP Fundamentals
Variables and Data Types
PHP is pretty laid back. It’ll take your word that you know what you’re doing with variable types:
$my_number = 42;
$my_text = "The answer to life, the universe, and everything.";
$my_array = array("PHP", "Fun", "Sometimes");
Control Structures
Decisions, decisions. PHP helps you make them:
if ($my_number > 100) {
echo "Wow, that's a lot!";
} else {
echo "Meh, seen bigger.";
}
Functions
Because nobody likes repeating themselves:
function greet($name) {
return "Hello, " . $name . "! How's PHP treating you?";
}
echo greet("You");
Diving Deeper
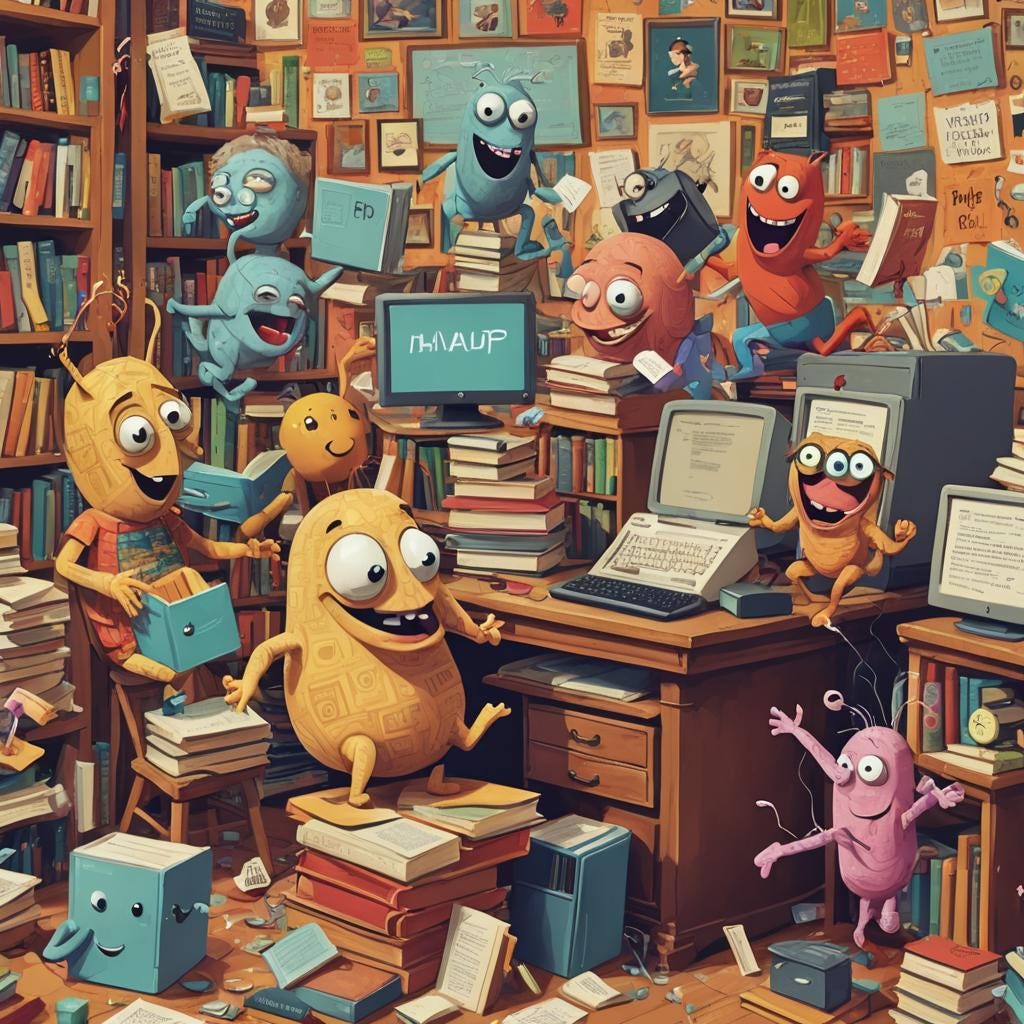
Working with Databases
PHP loves databases. They’re like best friends that share a love for organizing data:
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "myDB";
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
echo "Connected successfully";
Forms and User Input
PHP can handle form submissions with the elegance of a seasoned host:
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$name = htmlspecialchars($_POST["name"]);
echo "Welcome, " . $name . "!";
}
PHP’s Got Your Back (Security)
Remember, with great power comes great responsibility:
- Sanitize User Input: Always use
htmlspecialchars()
ormysqli_real_escape_string()
. - Validate Data: PHP gives you tools like
filter_var()
. - Password Hashing: Use
password_hash()
andpassword_verify()
for all your secret keeping needs.
The PHP Ecosystem
Beyond the basics, PHP has a vibrant ecosystem:
- Frameworks: Laravel, Symfony, CodeIgniter — pick your potion for building robust applications.
- CMS: WordPress, Drupal, Joomla — if you’re into content management, these are your playgrounds.
- APIs: Building web services? PHP with RESTful services is a match made in heaven.
Wrapping Up

PHP isn’t just for newbies. It’s a powerful tool that, with the right humor and a bit of patience, can help you create dynamic, robust web applications.
So, go forth, code with PHP, and remember — the PHP community is always here to laugh with you, not at you (most of the time). If you’ve got questions, comments, or just want to share your PHP journey, drop a comment below. Let’s make the PHP world a bit more amusing, one script at a time!
Stay curious, keep coding, and may the syntax be ever in your favor!