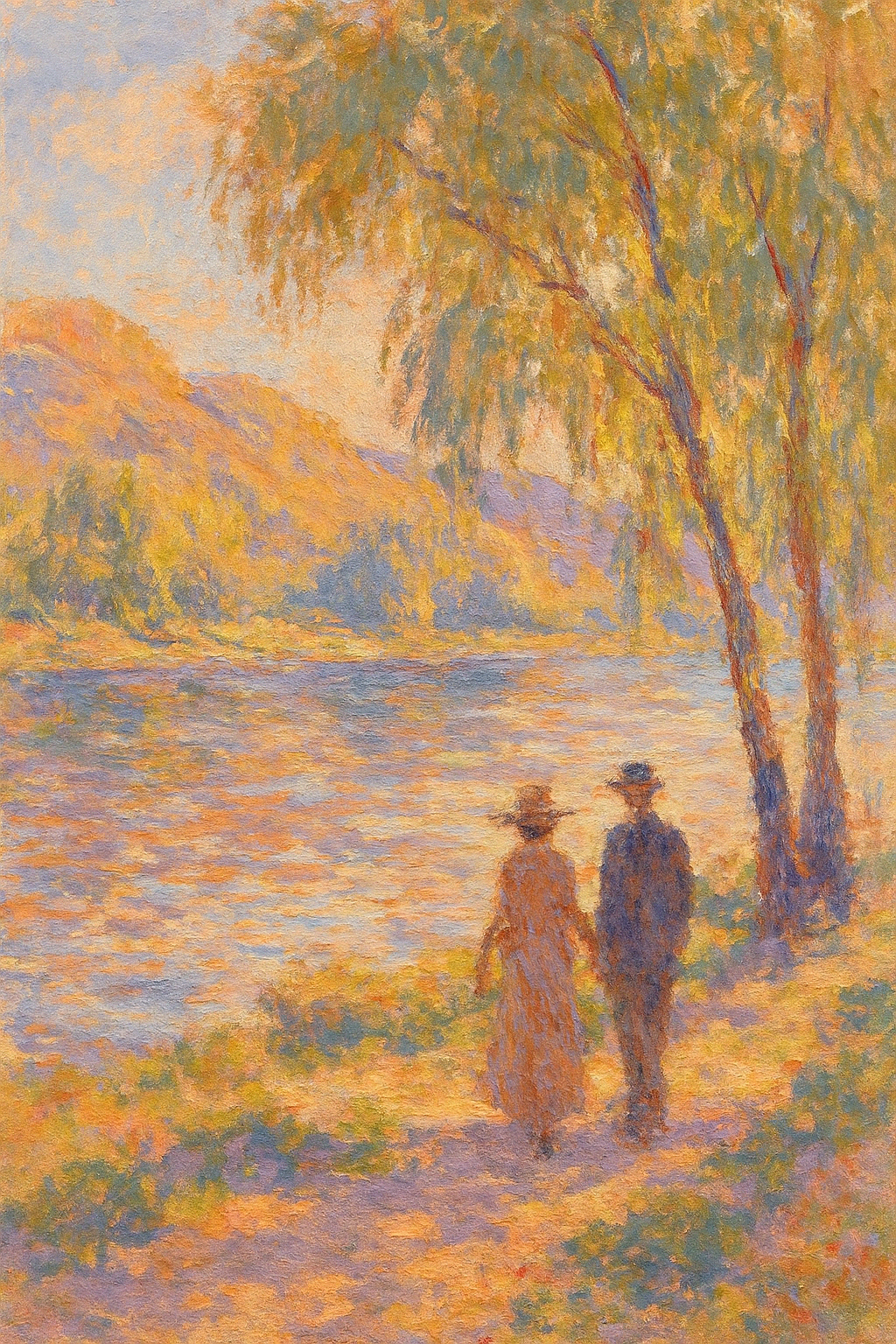
Once upon a time in the quirky land of Python, three ORMs walked into a bar. Django ORM, the well-dressed enterprise type. Peewee, the scrappy minimalist hipster. And Tortoise ORM, the async-savvy millennial with a nose for speed. What follows is their group therapy session… I mean, comparison guide.
Django ORM
What is it?
It’s the built-in ORM that comes with Django, the most popular web framework in Pythonland. Think of it as the high-functioning Type A personality of database abstraction.
Is it still relevant?
Absolutely. If you’re using Django, you’re using Django ORM. Resistance is futile.
Pros:
- Integrates deeply with Django (admin, forms, migrations).
- Mature and battle-tested.
- Huge community.
Cons:
- Coupled tightly with Django (try using it outside, and it throws a tantrum).
- Not async-friendly.
- Verbose when you’re trying to do complex queries.
Used for:
Everything Django — from personal blogs to giant enterprise web apps.
Example:
# Models
class Book(models.Model):
title = models.CharField(max_length=255)
published = models.DateField()
# Query
Book.objects.filter(title__icontains="Python")
Alternatives: Peewee, Tortoise, SQLAlchemy.
Subject of Famous Art?
Only if someone painted a surrealist nightmare of chained dependencies.
Popularity:
Still the king. Over 70k stars on GitHub. Strong StackOverflow presence.
https://github.com/django/django
Popularity Trend:
Flat but high. Like a pancake that never sags.
History & Inventor:
Developed alongside Django in 2005 by the Lawrence Journal-World team (yeah, a newspaper gave birth to Django).
Companies Using It:
Instagram (originally), Mozilla, Pinterest.
Similar To:
Rails’ ActiveRecord — but with more Python and less Ruby.
AI Compatibility:
Sure. It won’t help train your models, but it’ll serve the predictions like a butler with a silver tray.
Tech Stack:
Django, PostgreSQL, MySQL, SQLite. Works on Heroku, AWS, etc.
Best Tools:
Django Admin, Django REST Framework, Django Extensions.
Tidbit:
Every time you say “ORMs are slow,” a Django dev mutters “Use .select_related()
you fool!”
Peewee
What is it?
A small but mighty ORM that says “no thanks” to bloat and “yes please” to simplicity. Perfect for solo projects, embedded systems, or apps that aren’t trying to be Google.
Is it still relevant?
Very much. It’s the “IKEA furniture” of ORMs — affordable, quick, occasionally frustrating.
Pros:
- Lightweight and fast.
- Pure Python, no hard dependencies.
- Simple to learn.
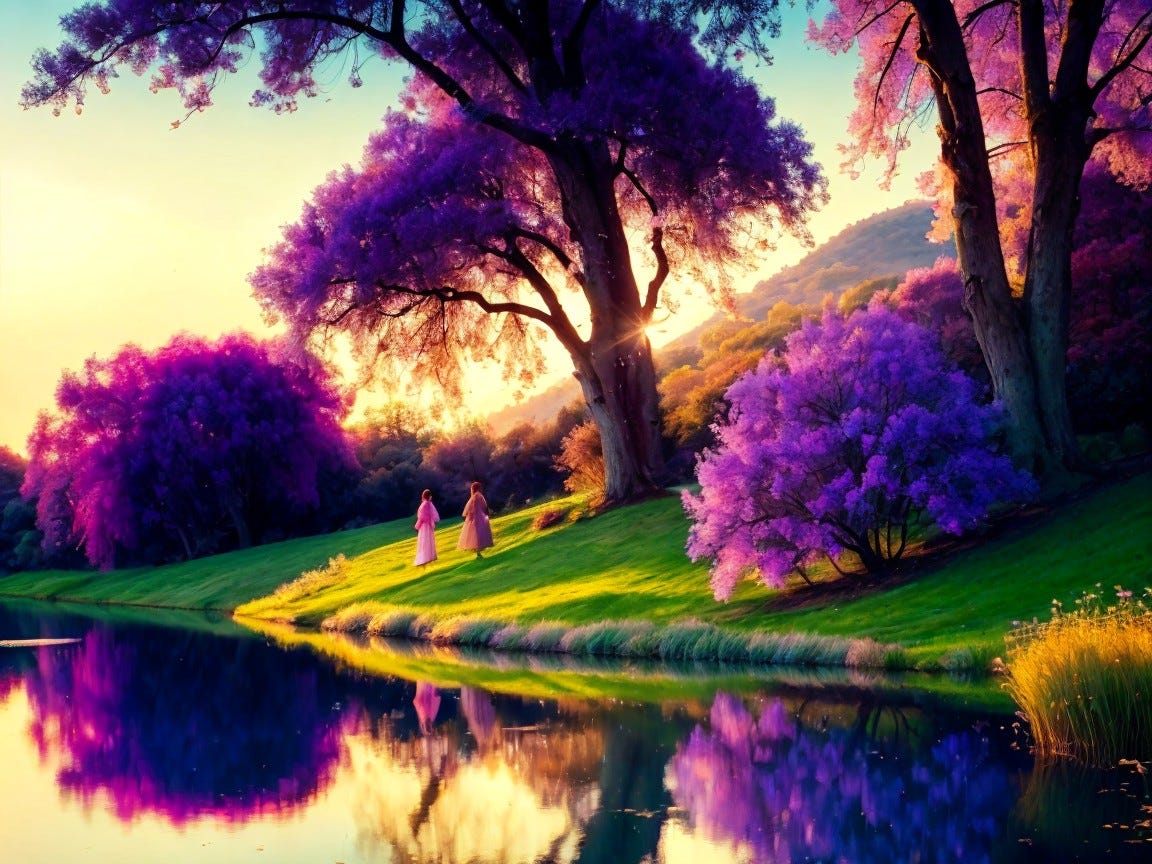
Cons:
- No async.
- Limited third-party ecosystem.
- Somewhat opinionated.
Used for:
CLI tools, microservices, bots, Raspberry Pi projects, or when Django feels like overkill.
Example:
# Models
class Book(Model):
title = CharField()
published = DateField()
# Query
Book.select().where(Book.title.contains("Python"))
Alternatives: SQLModel, dataset, raw SQL with regret.
Subject of Famous Art?
You could probably sketch Peewee in a cubist frame — compact blocks and strong lines.
Popularity:
Niche but loved. 10k+ GitHub stars.
https://github.com/coleifer/peewee
Trend:
Modest growth. Devs often discover it like a cool dive bar no one talks about.
History & Inventor:
Created by Charles Leifer in 2010. One dev, endless dedication.
Companies Using It:
Small-scale services, educational projects, indie apps.
Similar To:
SQLite for ORMs. Minimalist, functional, endearing.
AI Compatibility:
Good for backend storage in AI-powered bots or edge devices. No async, so not great for modern LLM-based APIs.
Tech Stack:
Works with SQLite, PostgreSQL, and MySQL.
Best Tools:
Peewee CLI, Playhouse extensions.
Tidbit:
Peewee can be fully explained in a single blog post. Try that with Django.
Tortoise ORM
What is it?
An asyncio-native ORM inspired by Django but built for the future — where await
is your best friend and synchronous code is so 2015.
Is it still relevant?
More than ever. Especially if you’re using FastAPI, Sanic, or any async-first framework.
Pros:
- Built for async Python.
- Django-style syntax without the baggage.
- Fast and efficient.
Cons:
- Not as mature as Django ORM.
- Smaller community.
- Debugging can feel like herding turtles.
Used for:
Modern APIs, real-time applications, async-first projects.
Example:
# Models
class Book(Model):
title = fields.CharField(max_length=255)
published = fields.DateField()
# Query
await Book.filter(title__icontains="Python").all()
Alternatives:
Gino, encode/databases, Beanie (for MongoDB).
Subject of Famous Art?
With a name like “Tortoise,” it’s begging for a surrealist painting of a giant turtle coded in async syntax.
Popularity:
Rising fast. Especially post-FastAPI boom.
https://github.com/tortoise/tortoise-orm
Trend:
Steady climb. It’s the up-and-comer your recruiter hasn’t heard of yet.
History & Inventor:
Spawned in 2018 by Chinese developer Zhenfeng Lei.
Companies Using It:
FastAPI users, hobbyists building async bots, and the kind of startups that say “microservice” unironically.
Similar To:
Django ORM on a hoverboard.
AI Compatibility:
Yes! Perfect for async APIs that chat with GPT or serve ML models in FastAPI.
Tech Stack:
Async frameworks (FastAPI, Starlette, Sanic), PostgreSQL, MySQL, SQLite.
Best Tools:
Aerich for migrations, FastAPI integration.
Tidbit:
Named after the Tortoise in “The Tortoise and the Hare,” reminding you that async doesn’t mean slow — it means patient and powerful.
Final Thoughts (and Friendly Nudges)
Every ORM here has its place. Django ORM is the well-rounded monolith. Peewee is the plucky indie. Tortoise ORM is the async trailblazer. Choose your fighter based on your use case, vibe, and caffeine level.
Let me know which one you prefer — and why.
Leave a comment, drop a tip, or just follow along for more snarky dev breakdowns.
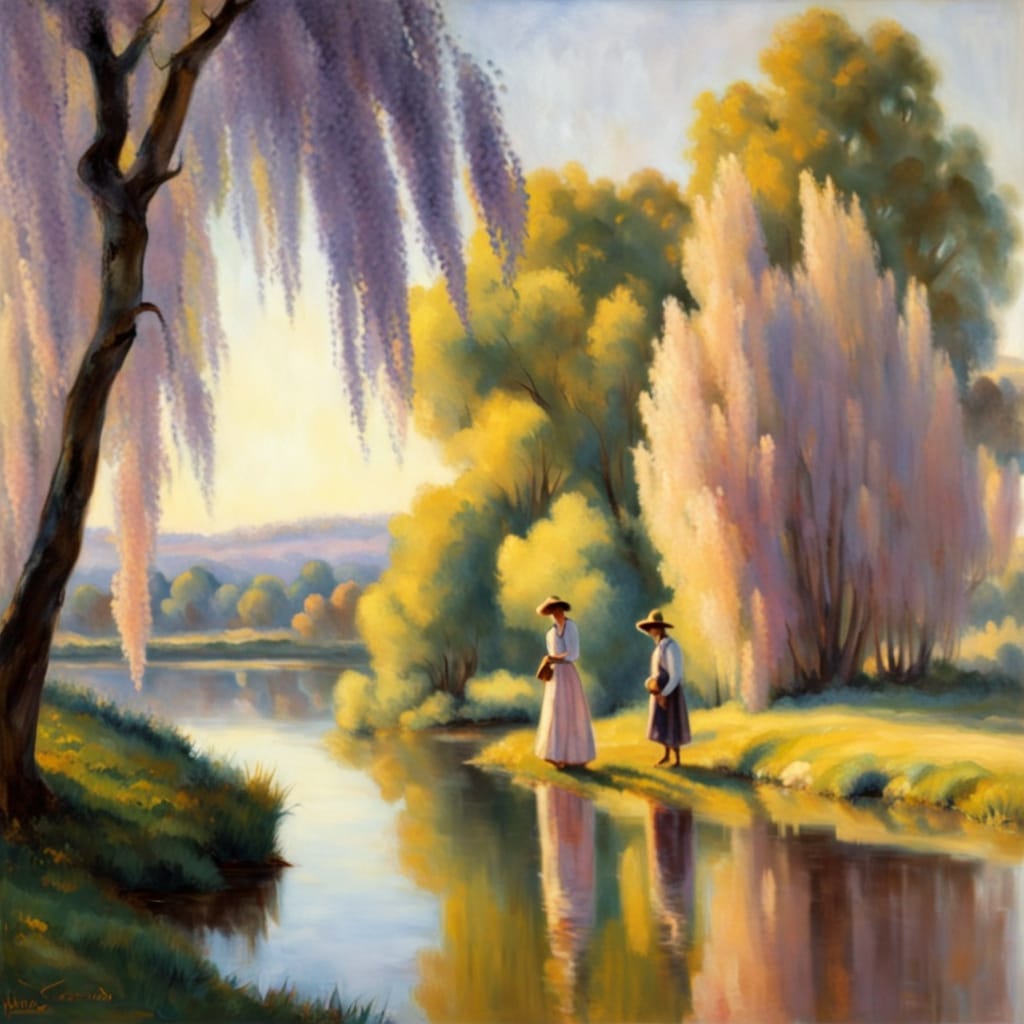
Art Prompt:
Gentle sun-dappled hills melt into a lake shimmering with pastel reflections. In the foreground, two figures stroll beneath willow trees, their forms softly blurred by the warm haze of late afternoon. The brushwork is loose and lively, capturing motion and light with delicate vibrancy. Use warm peach tones, soft lavender shadows, and powdery blue skies. The composition is open and airy, invoking the Impressionist elegance of late 19th-century French countryside scenes.