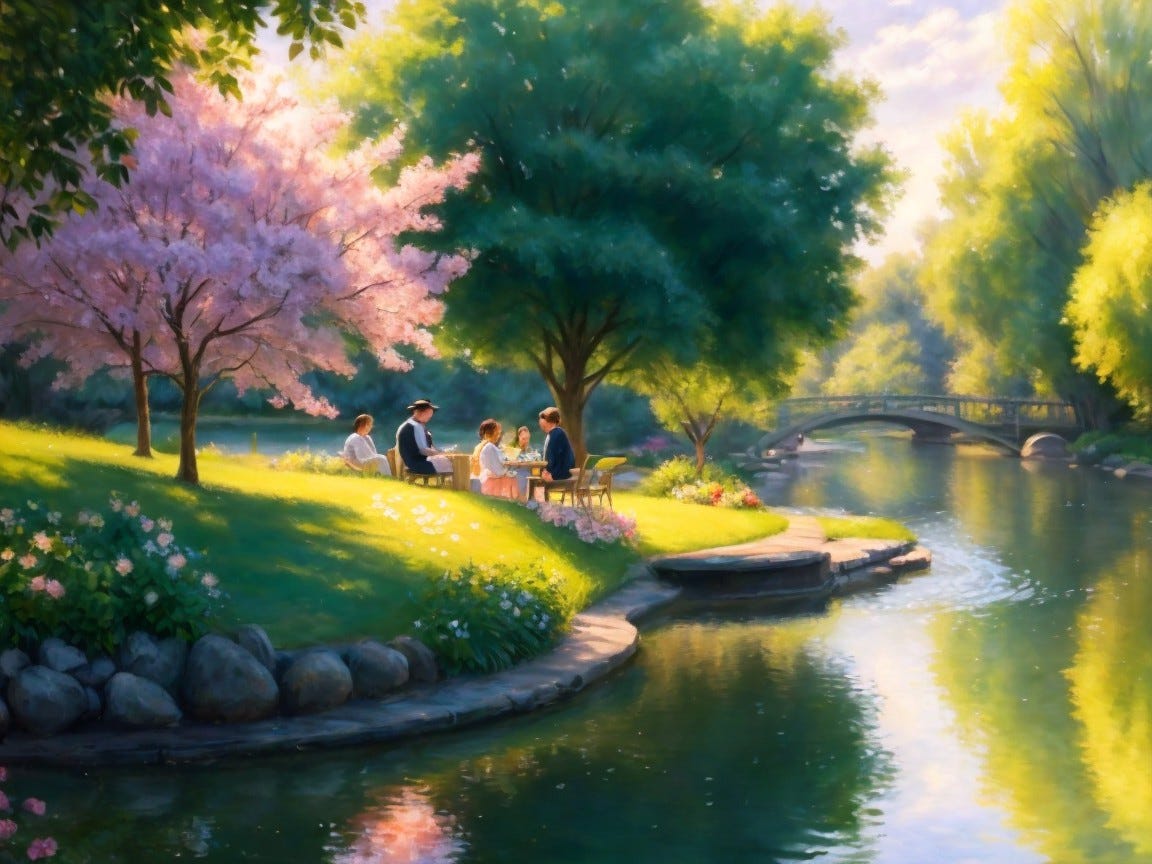
You know you’re a Python dev when “serialization” sounds less like data engineering and more like a Netflix original.
Today, let’s talk about three majestic beasts of the Python ecosystem that all do vaguely similar things, have wildly different personalities, and probably wouldn’t survive two hours on a group project together: Marshmallow, Attrs, and Django Models.
🐣 What is Marshmallow?
Marshmallow is a Python library for object serialization and deserialization, i.e., turning Python objects into JSON and back again — like a magical toaster that also makes espresso. It’s like the translator in a spy movie that keeps everyone from accidentally starting WW3.
- Relevant? Oh yeah. Especially if you’re not using Django.
- Strengths: Crazy powerful validation, pluggable fields, custom serialization, schema inheritance.
- Weaknesses: Slight learning curve; can feel verbose.
- Use Case: Cleanly separating your data schema from your business logic.
- Example:
from marshmallow import Schema, fields
class UserSchema(Schema):
name = fields.Str(required=True)
age = fields.Int()
UserSchema().load({"name": "Dave", "age": 9001})
- Alternatives: Pydantic (new hotness), Cerberus, Django Rest Framework Serializers.
- Famous Art Subject? Not yet, but if Picasso were a backend dev…
- Popularity: Stable. Often used in Flask apps and API microservices.
- Most Popular When? Around the rise of Flask and microservices architecture.
- History: Created by Steven Loria to make APIs suck less.
- Companies Using It: Many Flask-based platforms, internal tooling setups, and apps avoiding Django like a cat avoids water.
- AI Compatibility: Works well — especially with schema-driven generation or validation tools.
- Tech Stack Compatibility: Flask, FastAPI, bare Python.
- Best Tools With It: Flask, Connexion, apispec.
- Fun Tidbit: It can serialize to JSON, XML, YAML, and even alien languages if you’re feeling spicy.
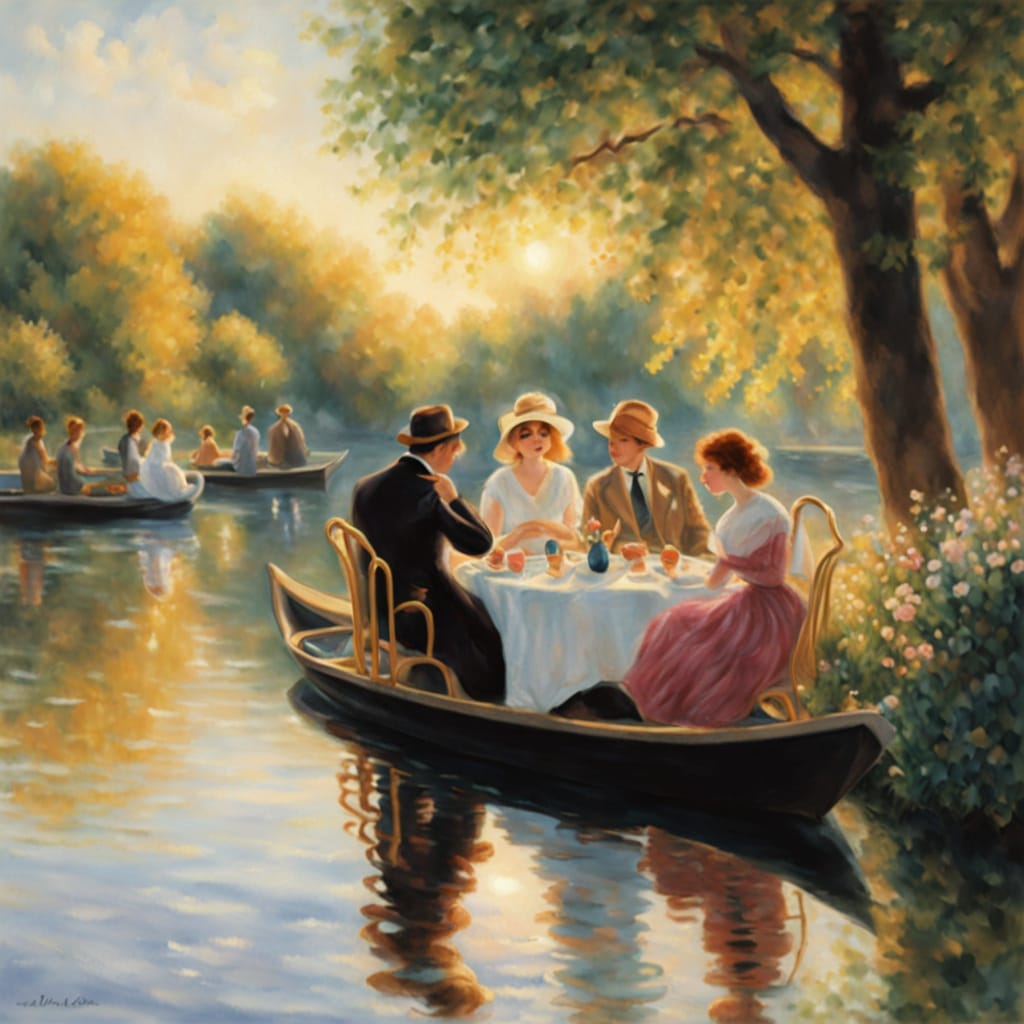
🧬 What is Attrs?
Attrs (short for “attributes”) is a delightful little library that makes writing classes in Python less soul-crushing. Think of it as dataclasses’ cool older cousin who rides a motorcycle and knows three types of encryption.
- Relevant? Still alive and kicking. Especially where control over behavior is key.
- Strengths: Immutability, validators, converters, hashability, and speed.
- Weaknesses: Slightly less beginner-friendly than
dataclasses
, and people keep forgetting to install it. - Use Case: When you want structured data objects with sane defaults and validation — without going full Django.
- Example:
import attr
@attr.s
class User:
name = attr.ib()
age = attr.ib(default=42)
User("Alice")
- Alternatives:
dataclasses
(built-in as of Python 3.7), Pydantic, plain ol’ classes. - Famous Art Subject? Michelangelo would’ve used it for his models… if he had Python 3.6.
- Popularity: Niche, but beloved in the right circles.
- Most Popular When? Pre-
dataclasses
era. Still solid for advanced uses. - History: Invented by Hynek Schlawack in a bid to free developers from boilerplate oppression.
- Companies Using It: Mozilla, Twisted, open source command-line tools.
- AI Compatibility: Great for structured data. Less common in AI-specific projects than Pydantic.
- Tech Stack Compatibility: Anything Pythonic.
- Best Tools With It: Hypothesis for property testing, cattrs for conversion, and your favorite IDE.
- Fun Tidbit: Once you start using it, you’ll question why you ever wrote
__init__
manually.
🧱 What is a Django Model?
Django Models are the Godzilla of Python data structures. Big. Powerful. Integrated with everything. And potentially destructive if mishandled. A Django Model is your data schema, ORM layer, and sometimes your therapist — all in one.
- Relevant? Absolutely. In Django, it is the way.
- Strengths: Integrated ORM, admin interface, migrations, robust querying.
- Weaknesses: Heavy; not fun outside Django; tightly coupled to database.
- Use Case: Web applications with relational databases that want to get stuff done fast.
- Example:
from django.db import models
class User(models.Model):
name = models.CharField(max_length=100)
age = models.IntegerField()
- Alternatives: SQLAlchemy, Tortoise ORM, Peewee.
- Famous Art Subject? Perhaps not, but they’re in a lot of nightmares.
- Popularity: Hugely popular — every Django app ever.
- Most Popular When? Perpetually. Django never dies.
- History: Part of Django since its beginning in 2005.
- Invented by: The Django team, originally Adrian Holovaty and Simon Willison.
- Companies Using It: Instagram, Pinterest, Mozilla, Bitbucket, and your favorite startup.
- AI Compatibility: Good enough, especially for AI-powered web apps with structured data.
- Tech Stack Compatibility: Django, Celery, PostgreSQL, Redis, and moral ambiguity.
- Best Tools With It: Django Admin, DRF, Graphene-Django.
- Fun Tidbit: Every time you use
objects.get_or_create()
, a junior dev levels up.
🎭 How Do They Compare?
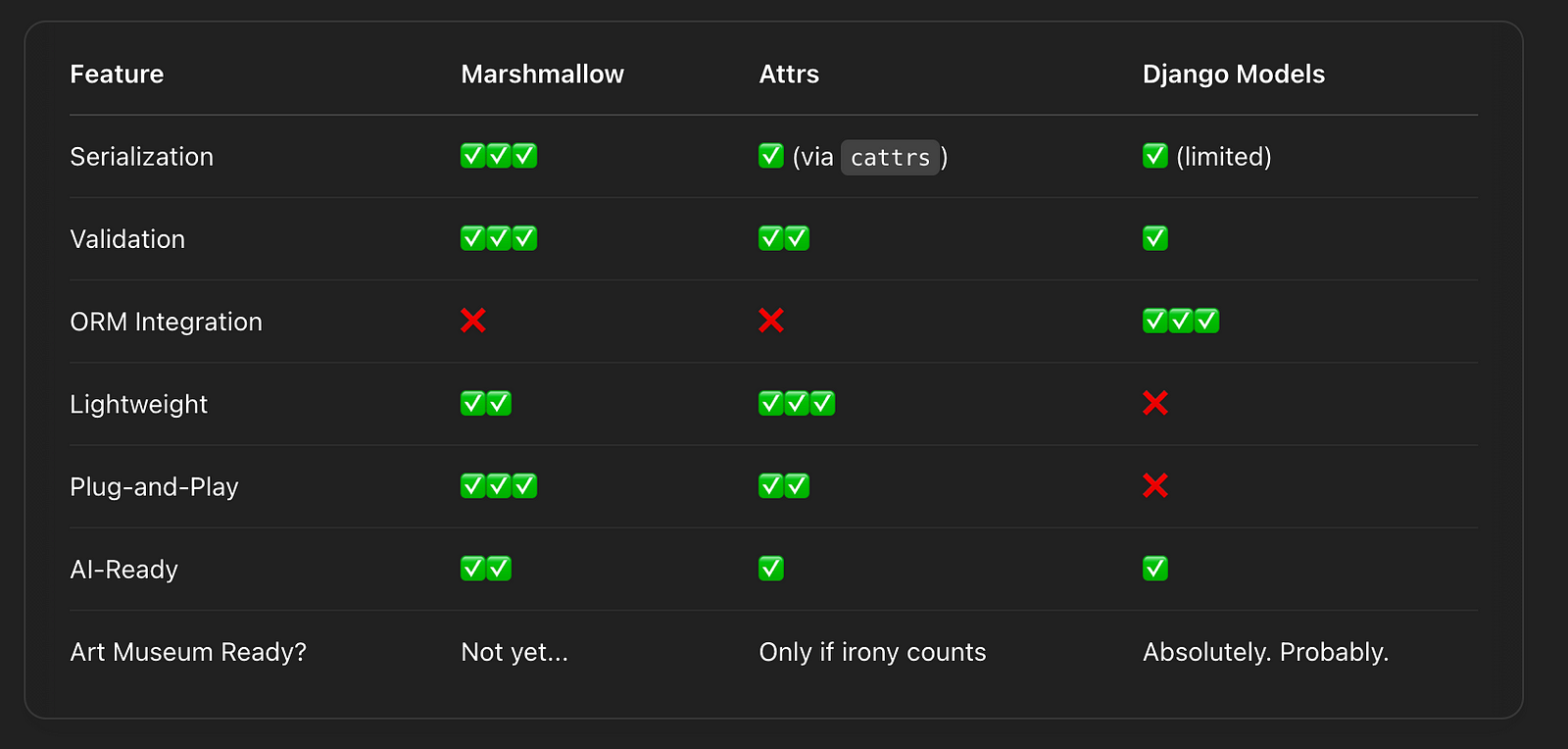
So Which One Should You Use?
- Building a clean API in Flask or FastAPI? Marshmallow is your friend.
- Making data classes without the constraints of Django? Attrs will make your life better.
- Doing web dev and enjoy automagic admin panels? Bow before Django Models.
And hey, sometimes you mix and match. There’s no rule saying you can’t use attrs
for your data layer and marshmallow
to serialize it while ignoring Django entirely and feeling smug about it.
Final Thoughts
Python gives you so many ways to define and work with data that it’s easy to go down a rabbit hole. The key is understanding the tradeoffs — and maybe keeping a cheat sheet on hand so you don’t wake up one morning wondering why your __init__
is 72 lines long.
If you made it this far, drop a comment. Which one do you swear by? Which one do you swear at?
And follow me for more nonsense like this. There’s always more nonsense.
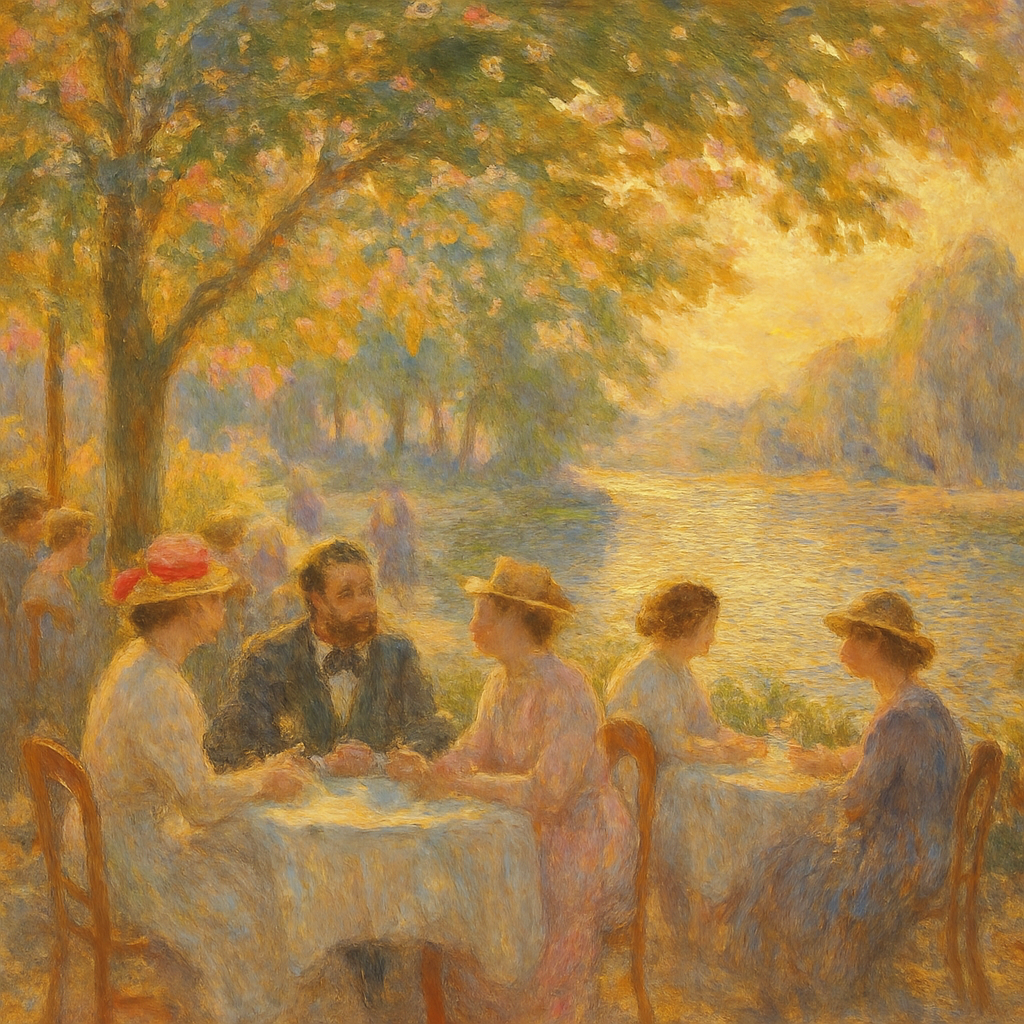
Art Prompt:
A sun-dappled riverside café scene, rendered with warm pastels and gentle brush strokes reminiscent of Renoir, capturing the fleeting beauty of a lazy summer afternoon. The composition features elegantly dressed figures in light conversation, surrounded by blossoming trees and flickering reflections on the water. The atmosphere is serene and luminous, suffused with a golden-hour glow that softens all edges and invites a quiet nostalgia.